1. continue
語法的基本理解
continue
語法用於 Python 的迴圈結構中,當滿足特定條件時,會跳過該次迭代並進入下一次迭代。這在需要排除特定元素的處理時非常實用。
1.1 continue
的基本語法
continue
語法可用於for
迴圈或while
迴圈中。基本語法如下:
for i in range(5):
if i == 2:
continue
print(i)
在這段程式碼中,當i
等於2時會執行continue
,因此print
語句將被跳過,輸出結果為0, 1, 3, 4
。
2. 在for
迴圈中使用continue
for
迴圈通常用於重複處理數據。在這種情況下,使用continue
可以根據特定條件跳過該次迭代的剩餘處理。
2.1 基本的for
迴圈範例
以下程式碼依序處理列表中的數值,並根據條件跳過特定處理:
numbers = [1, 2, 3, 4, 5]
for num in numbers:
if num % 2 == 0:
continue
print(num)
此程式碼中,偶數會被continue
跳過,因此輸出為1, 3, 5
。
2.2 巢狀for
迴圈中的continue
在巢狀迴圈中使用continue
時,它只會影響內層的迴圈。例如:
for i in range(3):
for j in range(3):
if j == 1:
continue
print(i, j)
在此程式碼中,當內層迴圈的j
等於1時,執行continue
並跳過處理。因此輸出結果為(0, 0), (0, 2), (1, 0), (1, 2), (2, 0), (2, 2)
。
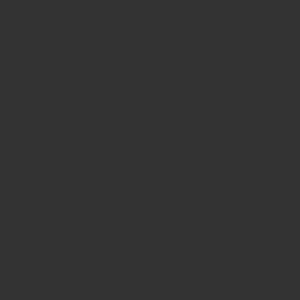
3. 在while
迴圈中使用continue
在while
迴圈中同樣可以使用continue
來跳過當前的迭代,進入下一次迭代。
3.1 基本的while
迴圈範例
以下程式碼讓使用者輸入數值,並根據特定條件使用continue
跳過處理。
counter = 0
while counter < 5:
counter += 1
if counter == 3:
continue
print(counter)
此程式碼中,當counter
等於3時執行continue
,跳過print
語句。因此輸出為1, 2, 4, 5
。
4. continue
與else
的組合
在 Python 中,可以將for
或while
迴圈與else
區塊組合使用。即使執行了continue
,else
區塊仍會執行,使流程控制更加靈活。
4.1 使用else
區塊的範例
以下範例將else
與continue
組合,執行迴圈結束後的處理:
for i in range(3):
for j in range(3):
if j == 1:
continue
print(i, j)
else:
print("內層迴圈完成。")
即使在內層迴圈中執行了continue
,else
區塊的”內層迴圈完成。”仍會顯示。
5. continue
與break
的區別
continue
與break
都是用來控制迴圈流程的語法,但用途不同。
5.1 continue
的作用
continue
會跳過當前的迭代並進入下一次迭代,不會終止整個迴圈。
5.2 break
的作用
相反地,break
會直接結束整個迴圈並跳出迴圈區塊。以下範例展示兩者的區別:
for i in range(5):
if i == 3:
break
print(i)
此程式碼中,當i
等於3時執行break
,整個迴圈結束。因此輸出為0, 1, 2
。
5.3 應該使用哪一個?
continue
適用於根據條件跳過某些處理,而break
則適用於直接終止迴圈。請根據具體需求選擇合適的語法。
6. 實際應用範例
continue
語法在實際編程中非常有用,尤其是用於提高程式效率或避免特定情況。
6.1 資料過濾
例如,可以使用continue
從資料集中排除特定值:
data = [1, -1, 2, -2, 3, -3]
for value in data:
if value < 0:
continue
print(value)
此程式碼會跳過負數,因此輸出為1, 2, 3
。
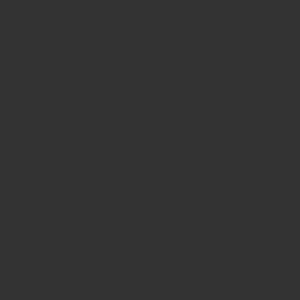
7. 常見錯誤與故障排除
以下將介紹使用continue
時常見的錯誤及其解決方法。
7.1 縮排錯誤
使用continue
最常見的錯誤是縮排不正確。如果continue
被錯誤縮排,可能會導致預期外的行為。
7.2 無限迴圈的風險
在while
迴圈中使用continue
時,必須確保迴圈的條件能夠更新,否則可能會進入無限迴圈。例如:
counter = 0
while counter < 5:
if counter == 3:
continue # 導致無限迴圈
counter += 1
print(counter)
此程式碼會因continue
跳過counter
的更新,而導致無限迴圈。
8. 結論
continue
語法是 Python 中用來控制迴圈流程的強大工具。它能夠幫助您靈活地處理資料,跳過不必要的步驟,並提高程式的可讀性與效率。
透過本教學內容,您應該已經掌握了continue
的基本語法、for
與while
中的應用、以及與break
的區別。請根據具體需求靈活使用,以優化程式邏輯。