- 1 1. Role of the pass Statement in Python
- 2 2. What is the pass Statement?
- 3 3. Situations Where the pass Statement is Used
- 4 4. Difference Between pass, continue, and break Statements
- 5 5. Common Misunderstandings of the pass Statement and How to Avoid Errors
- 6 6. Summary: Effective Use of the pass Statement
1. Role of the pass Statement in Python
Python is known for allowing developers to write clean and readable code compared to many other programming languages. Among its features, the “pass statement” plays a particularly unique role in Python. Typically, when writing code, you are required to include some processing logic, but the pass statement is an exception. It is used to explicitly indicate that “nothing is to be done.”
For example, when creating functions or classes, if you haven’t yet decided on the internal processing logic or plan to add functionality later, you can use the pass statement to avoid errors while creating the skeleton of the code. Additionally, it is used in conditional branches or loops to indicate “do nothing.”
Thus, the pass statement is an extremely useful feature in Python for expressing the intent of “pending” or “unimplemented” sections within code. This article will provide a detailed explanation of the pass statement’s usage and its practical applications.
2. What is the pass Statement?
The pass statement in Python is used to explicitly signify “doing nothing.” Python code typically requires some processing logic, but there are situations where you want to delay processing or temporarily avoid executing anything. In these cases, the pass statement helps prevent errors while postponing future actions.
Basic Syntax of the pass Statement
def my_function():
pass
In this example, the function my_function()
is defined, but there is no processing inside the function. However, by using the pass statement, you can clearly indicate that “this function is unimplemented, but should not raise an error.”
Example: Placeholders for Classes or Functions
In the early stages of development, it’s common to create the basic structure of the code. Often, the actual processing is added later, and the pass statement is used to initially build the structure.
class MyClass:
pass
In this case, the class MyClass
has not been implemented yet, but syntactically, the code is valid. The pass statement marks the unimplemented section, enabling development to proceed without errors.
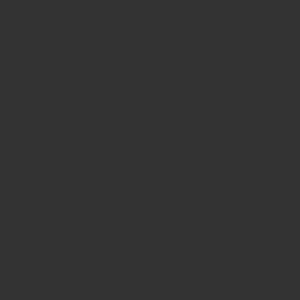
3. Situations Where the pass Statement is Used
The pass statement is useful in various situations. It is particularly effective in the following cases:
1. Unimplemented Functions or Classes
As mentioned earlier, when creating functions or classes where the internal implementation has not yet been decided, the pass statement is used. This prevents errors before the code is fully developed and ensures smooth progression when adding implementations later.
def future_function():
pass
2. Using pass in the else Clause of Conditional Statements
Sometimes, in conditional statements like an else clause, you may want to do nothing for certain conditions. Since Python will raise an error if there is no processing in the if or else statements, the pass statement is used to avoid errors.
for i in range(5):
if i % 2 == 0:
print(i)
else:
pass # Do nothing for non-even numbers
3. Used in Exception Handling
In some cases, when an exception occurs, you might not want to take any action. In such cases, the pass statement is commonly used. It allows the program to continue running even when an exception occurs.
try:
risky_function()
except SomeError:
pass # Do nothing if an error occurs
4. Difference Between pass, continue, and break Statements
The pass statement means “do nothing,” but Python also has other control statements, each serving a different purpose. Here, we’ll explain the differences between the pass, continue, and break statements.
Difference Between pass and continue Statements
The continue statement is used to skip the current iteration of a loop and move to the next one. In contrast to pass, which does nothing, the continue statement moves the loop to the next iteration.
for i in range(5):
if i % 2 == 0:
continue # Skip even numbers
print(i) # Only odd numbers are printed
Difference Between pass and break Statements
The break statement is used to terminate a loop early. Unlike pass and continue, which modify the loop’s execution in different ways, break completely ends the loop and moves to the next part of the code.
for i in range(5):
if i == 3:
break # Exit the loop when reaching 3
print(i)
In contrast, pass does nothing and simply continues with the loop or conditional statement as normal. Each of these control statements serves a distinct purpose.
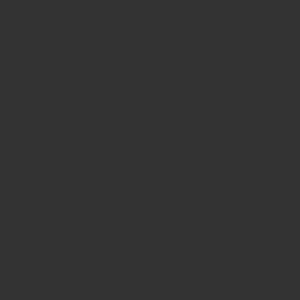
5. Common Misunderstandings of the pass Statement and How to Avoid Errors
A common mistake for beginners in Python is using the pass statement in situations where it’s not needed. The pass statement should only be used in special cases where “a statement is required, but nothing needs to be done.”
Using pass to Avoid Errors
For example, if the processing in an if statement is undecided, an error will occur. In such cases, the pass statement can be used to avoid errors.
a = 10
if a < 5:
pass # Using pass to avoid errors while deciding on processing
else:
print("a is greater than or equal to 5")
Similarly, when defining classes or functions, using pass allows you to indicate that “the implementation will come later” while avoiding errors.
6. Summary: Effective Use of the pass Statement
The pass statement plays a unique role in Python by allowing you to “do nothing.” It is mainly used in unimplemented functions, classes, conditional statements, and exception handling to avoid errors and keep code moving forward.
Unlike other control statements like continue and break, the pass statement doesn’t involve skipping iterations or terminating loops—it simply does nothing. Understanding the correct usage of the pass statement will help you efficiently progress in development, making it a valuable tool for both beginners and experienced developers alike.