- 1 1. Introduction
- 2 2. Basic Usage of the find() Method
- 3 3. Advanced Usage of the find() Method
- 4 4. Difference between find() and rfind()
- 5 5. Error Handling and Comparison with the index() Method
- 6 6. Using with Regular Expressions (Advanced Usage)
- 7 7. Summary and Practical Examples
- 8 8. Frequently Asked Questions (FAQ)
1. Introduction
Python offers numerous string manipulation methods, among which the find()
method is a highly useful function for locating a specific substring and obtaining its position. It scans the string from the start to the end and returns the index of the first matching substring. In this article, we will gradually explain the basic usage of the find()
method, its advanced use cases, as well as related methods such as rfind()
and error handling.
Why is the find()
Method Important?
Using the find()
method can significantly improve the efficiency of string searching in Python programs. This method is especially helpful when dealing with large amounts of text data or log files, as it allows for easy retrieval of the desired information.
Additionally, it is beginner-friendly and frequently used in programming, making it crucial to understand as a foundation for string manipulation in Python.
2. Basic Usage of the find()
Method
Basic Syntax of the find()
Method
First, let’s look at the basic usage of the find()
method. Below is its syntax:
str.find(sub[, start[, end]])
sub
: The substring to search forstart
: The index to start searching (optional)end
: The index to stop searching (optional)
The find()
method returns the index of where the specified substring is located within the string. If the substring is not found, it returns -1
.
Basic Example
Let’s look at a concrete example:
text = "Hello, Python!"
result = text.find("Python")
print(result) ## Output: 7
In this example, the substring "Python"
is found at index 7 in the original string, so the result returned is 7
.
Searching in a Subrange
The find()
method also allows you to specify a start
and end
argument, which limits the search to a specific range within the string.
text = "Hello, Python!"
result = text.find("Python", 0, 5)
print(result) ## Output: -1
In this case, the search is limited to the range from start=0
to end=5
, but the substring "Python"
is not found, so -1
is returned.
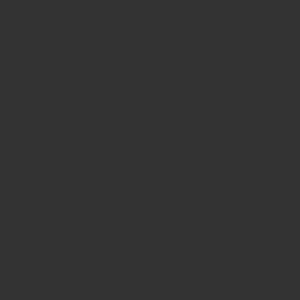
3. Advanced Usage of the find()
Method
Multiple Searches
By using the find()
method in a loop, you can retrieve all the indices of a substring when it appears multiple times within a string.
text = "Python is great, and Python is powerful."
index = text.find("Python")
while index != -1:
print(f"'Python' found at index {index}")
index = text.find("Python", index + 1)
In this example, since Python
appears multiple times, all positions will be printed.
Searching within a Specific Range
If you want to search for a substring within a specific range, you can use the start
and end
arguments to limit the search range.
text = "A quick brown fox jumps over the lazy dog."
result = text.find("quick", 2, 10)
print(result) ## Output: 2
In this case, the substring "quick"
is found between start=2
and end=10
, and the starting index is returned.
4. Difference between find()
and rfind()
Introduction to the rfind()
Method
Another method similar to find()
is rfind()
. The rfind()
method searches for a substring starting from the end of the string and returns the index of the first matching position. The syntax is the same as find()
, but the search direction is different.
text = "Hello, world!"
result = text.rfind("o")
print(result) ## Output: 8
In this example, the substring "o"
is searched from the end of the string, and the first occurrence at index 8 is returned.
When to Use find()
vs rfind()
It is important to choose between find()
and rfind()
depending on the use case. For instance, when analyzing log files or text data, if you need to find the last occurrence of a particular string, rfind()
is useful.
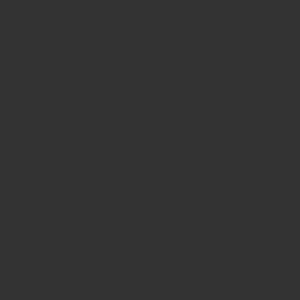
5. Error Handling and Comparison with the index()
Method
Difference between find()
and index()
Another method similar to find()
is index()
. The difference is that find()
returns -1
when the substring is not found, whereas index()
raises an error (ValueError
).
text = "Hello, Python!"
try:
result = text.index("Java")
except ValueError:
print("Substring not found.")
In this example, since the substring "Java"
is not found, a ValueError
is raised and handled using a try-except
block.
Importance of Error Handling
When handling user input in programs, it is crucial to provide appropriate error handling for unexpected inputs. The find()
method allows flexible string searching while avoiding errors.
6. Using with Regular Expressions (Advanced Usage)
Using with re.search()
By using regular expressions, more complex patterns can be searched within strings. Python’s re
module has the re.search()
function, which can be used to search for patterns using regular expressions.
import re
text = "Hello, Python!"
match = re.search(r"bPw+", text)
if match:
print(match.group()) ## Output: Python
In this example, a substring starting with the letter “P” is searched and returned if it matches.
Advantages of Regular Expressions
The find()
method is efficient for simple searches, but using regular expressions allows for more advanced pattern matching. For instance, regular expressions are very useful when searching for email addresses or phone numbers.
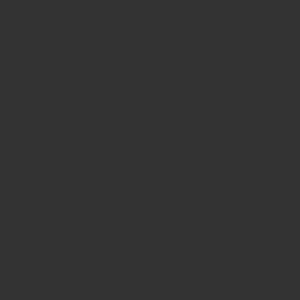
7. Summary and Practical Examples
Summary
In this article, we covered the basic and advanced usage of Python’s find()
method, along with its differences from the related rfind()
and index()
methods. The find()
method is a simple yet powerful tool for string searching.
Practical Usage Example
In real-world projects, the find()
method is frequently used for log analysis and large-scale text data processing. For example, it can be used in the following scenario:
log_data = "2024-10-05: Error occurred in module XYZ"
if log_data.find("Error") != -1:
print("An error occurred.")
Using the find()
method in this way allows for efficient extraction of important information from text data.
8. Frequently Asked Questions (FAQ)
What is the difference between find()
and the in
operator?
In Python, both the find()
method and the in
operator can be used for string searching. The key difference lies in their return values.
find()
method: Returns the starting index of the substring if found. Returns-1
if not found.
text = "Hello, Python!"
index = text.find("Python")
print(index) ## Output: 7
in
operator: ReturnsTrue
orFalse
indicating whether the substring exists. The position is not returned.
text = "Hello, Python!"
exists = "Python" in text
print(exists) ## Output: True
Can find()
be used on a list?
No, the find()
method is only available for strings. If you want to search through a list or other data structures, use the in
operator or the index()
method.
- Example: Checking if an element is present in a list.
my_list = [10, 20, 30, 40]
if 20 in my_list:
print("20 is in the list.")
- Example: Finding the position of an element in the list.
my_list = [10, 20, 30, 40]
index = my_list.index(20)
print(index) ## Output: 1
How should I choose between find()
and regular expressions?
The find()
method is very useful for simple string searches. However, when you need to perform more complex pattern-based searches, using regular expressions is more effective. For example, regular expressions are ideal for searching for specific formats like email addresses or dates.
- Example: Searching for email addresses using regular expressions.
import re
text = "Please contact us at support@example.com"
match = re.search(r'b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+.[A-Z|a-z]{2,}b', text)
if match:
print(f"Found email: {match.group()}")
Depending on the situation, using find()
and regular expressions can be very effective for your needs.