1. Basic Usage of Python’s abs() Function
What is the abs() function in Python for calculating absolute values?
The abs()
function in Python is a built-in function that returns the absolute value of a given number. The absolute value refers to the magnitude of the number, ignoring its sign (positive or negative). The abs()
function is very simple and can be used with integers, floating-point numbers, and even complex numbers.
Basic Usage
The usage of the abs()
function is very intuitive. You simply pass a number as an argument, and it returns its absolute value. Below is a specific example:
# Example for integers
x = -10
y = abs(x)
print(y) # Output: 10
# Example for floating-point numbers
a = -3.14
b = abs(a)
print(b) # Output: 3.14
# Example for complex numbers
z = 3 + 4j
w = abs(z)
print(w) # Output: 5.0
By using the abs()
function, you can easily calculate the absolute value of a number. In particular, for complex numbers, the absolute value is computed based on the Pythagorean theorem, making it very useful for mathematical calculations.
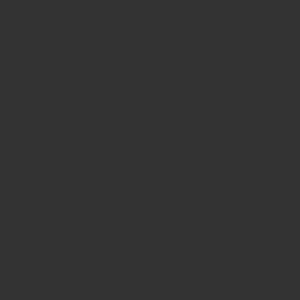
2. Difference Between abs() and math.fabs() Functions
What is math.fabs()?
The math
module in Python’s standard library provides the fabs()
function, which also returns the absolute value, similar to abs()
. This function takes a real number (either an integer or a floating-point number) as an argument and always returns the result as a floating-point number.
Differences Between abs() and math.fabs()
The main difference between the abs()
function and math.fabs()
lies in the data type of the return value. The abs()
function returns an integer if the argument is an integer, or a floating-point number if the argument is a floating-point number. On the other hand, math.fabs()
always returns a floating-point number.
import math
# Comparing abs() and math.fabs()
x = -10
print(abs(x)) # Output: 10 (integer)
print(math.fabs(x)) # Output: 10.0 (floating-point)
y = -3.14
print(abs(y)) # Output: 3.14 (floating-point)
print(math.fabs(y)) # Output: 3.14 (floating-point)
math.fabs()
always returns a floating-point number, so it is useful when you want to handle all results as floating-point numbers. It is especially beneficial in situations where consistency in data types is required during numerical processing. In these cases, math.fabs()
is a more suitable option.
3. Calculating Absolute Values for Arrays and Lists
Limitations of abs() and Using NumPy
The abs()
and math.fabs()
functions are generally used for individual numbers. They cannot be directly applied to lists or arrays to calculate the absolute values of all elements at once. However, by using the NumPy library, you can efficiently calculate the absolute values for elements in a list or array.
NumPy’s abs() Function
NumPy has a function called np.abs()
, which can be used to calculate the absolute value for all elements in a list or array.
import numpy as np
# Calculating absolute values for an array
arr = np.array([-1, -2, -3, 4])
abs_arr = np.abs(arr)
print(abs_arr) # Output: [1 2 3 4]
Additionally, NumPy supports arrays that contain complex numbers, allowing you to compute the absolute values of complex numbers all at once.
# Absolute values for an array of complex numbers
complex_arr = np.array([3 + 4j, 1 - 1j])
abs_complex_arr = np.abs(complex_arr)
print(abs_complex_arr) # Output: [5. 1.41421356]
Absolute Value Calculation with pandas
If you want to calculate the absolute values of specific columns in a DataFrame, you can use the DataFrame.abs()
method from the pandas library.
import pandas as pd
# Absolute value calculation for a DataFrame
df = pd.DataFrame({'A': [-1, -2, -3], 'B': [4, -5, 6]})
print(df.abs())
# Output:
# A B
# 0 1 4
# 1 2 5
# 2 3 6
By leveraging NumPy and pandas, you can efficiently calculate the absolute values for large datasets, making it useful for data processing and analysis tasks.
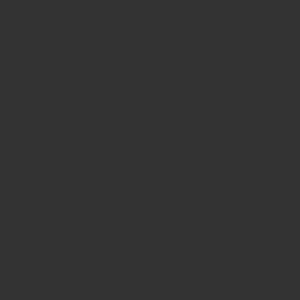
4. Practical Use Cases
Example 1: Calculating Absolute Values for Error Data
In data analysis, absolute values are often used to measure the magnitude of errors. For instance, you can calculate the absolute error between experimental results and theoretical values, and by averaging these errors, you can assess the variation and stability of the data.
# Calculating absolute values for error data
errors = [1.5, -2.3, 0.9, -1.2, 0.4]
abs_errors = [abs(err) for err in errors]
# Calculating the mean absolute error
average_abs_error = sum(abs_errors) / len(abs_errors)
print(average_abs_error) # Output: 1.26
In the above code, the absolute values of the errors are calculated using list comprehension, and the mean error is computed. This approach allows you to evaluate errors based solely on their magnitude, regardless of whether they are positive or negative.
Example 2: Using Absolute Value for Complex Numbers
The absolute value of a complex number represents its magnitude (or amplitude) and is frequently used in fields like physics and engineering. For example, the absolute value of a complex number is crucial in electrical impedance calculations or vibration analysis.
# Calculating the absolute value of a complex number
z = 3 + 4j
z_abs = abs(z)
print(z_abs) # Output: 5.0
The absolute value of a complex number is calculated based on the Pythagorean theorem, deriving its length (magnitude) from the x and y axis components. This calculation is helpful in signal processing and physical simulations to assess amplitude.
Example 3: Applying Absolute Value Calculation in Data Analysis
Calculating the absolute value of data in a DataFrame is useful for data cleaning and detecting outliers. For example, by calculating the absolute value of normalized datasets, you can visualize extremely large negative or positive values and analyze them as part of your analysis.
import pandas as pd
# Calculating the absolute values of differences in a DataFrame
data = {'Experimental Results': [-5, 3, -2, 8, -7], 'Predicted Values': [5, 3, 2, 8, 7]}
df = pd.DataFrame(data)
df['Absolute Difference'] = (df['Experimental Results'] - df['Predicted Values']).abs()
print(df)
# Output:
# Experimental Results Predicted Values Absolute Difference
# 0 -5 5 10
# 1 3 3 0
# 2 -2 2 4
# 3 8 8 0
# 4 -7 7 14
In the example above, the absolute difference between experimental results and predicted values is calculated, making it easier to visualize and identify outliers. This approach is especially useful for evaluating machine learning models or during data cleaning tasks.
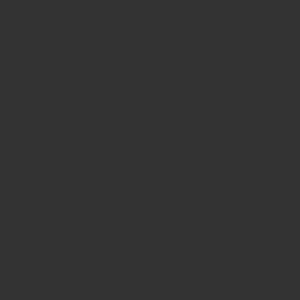
5. Other Considerations and Best Practices
Error Handling in Absolute Value Calculations
The abs()
function is very simple and works well for integers, floating-point numbers, and complex numbers. However, if you try to apply it to data types like lists or dictionaries, errors will occur. Therefore, it is recommended to check the data type or add proper exception handling before calculating the absolute value in your code.
# Absolute value calculation with error handling
def safe_abs(value):
try:
return abs(value)
except TypeError:
print(f"TypeError: {value} is a type that cannot calculate the absolute value.")
return None
print(safe_abs([-1, -2])) # Output: TypeError: [-1, -2] is a type that cannot calculate the absolute value.
Performance Considerations
When calculating absolute values for large amounts of data, built-in functions like abs()
and math.fabs()
are efficient. However, for large datasets, using NumPy or pandas can be more efficient. This can improve processing speed and allow you to handle large calculations without sacrificing performance.
Making the Right Choice: abs(), math.fabs(), or numpy.abs()
It is important to choose the right function based on the situation:
abs()
is ideal for small-scale calculations.math.fabs()
is suitable when you want to treat all numbers as floating-point numbers.numpy.abs()
orpandas.DataFrame.abs()
should be used for calculations on lists, arrays, or data frames, as they provide better performance for large datasets.
6. Conclusion
In this article, we have explored the abs()
function in Python, focusing on how to calculate absolute values and its practical applications. The calculation of absolute values is extremely useful in handling numerical data, performing analysis, and error handling. By correctly utilizing functions like abs()
, math.fabs()
, and numpy.abs()
, you can significantly improve the efficiency of your programming tasks.
By understanding how to calculate absolute values in Python, you can apply it to real-world projects and analyses, writing more powerful and efficient code.