1. What is YAML?
Overview of YAML
YAML (YAML Ain’t Markup Language) is a data serialization format widely used to represent structured data. It is similar to JSON and XML, but its main features are simplicity and high readability. One of the key advantages is that it uses indentation to represent hierarchical structures, making it more human-readable.
Differences from JSON and XML
JSON and XML are also formats used to describe data, but compared to these, YAML is less verbose, simpler, and easier to understand. For example, JSON uses curly braces {}
and commas ,
frequently, which can make it harder to read, especially when dealing with large data sets. On the other hand, YAML uses indentation to show structure, making it visually easier to understand the data hierarchy.
Compatibility with Python
The syntax of Python, which uses indentation to define blocks, is naturally compatible with the YAML format. Furthermore, by using the Python library “PyYAML,” you can easily read and write YAML files, making it a popular choice for configuration files.
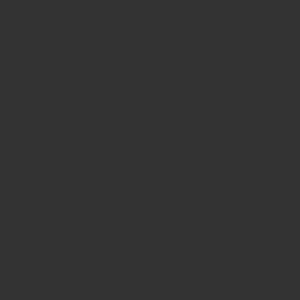
2. How to Read and Write YAML Files in Python
Reading YAML Files
To read YAML files in Python, you first need to install the “PyYAML” library and use the yaml.safe_load()
function. This function safely converts YAML data into Python dictionaries and lists. Below is an example of basic reading.
import yaml
# Open the YAML file and read its contents
with open('config.yaml', 'r') as file:
data = yaml.safe_load(file)
print(data)
This code reads data from a YAML file and treats it as a Python dictionary. For example, if you read the following YAML file:
database:
host: localhost
port: 3306
In Python, the dictionary would look like this:
{'database': {'host': 'localhost', 'port': 3306}}
Writing YAML Files
To write Python data into a YAML format, you use the yaml.dump()
function. The following example writes a Python dictionary to a YAML file.
import yaml
data = {
'name': 'John Doe',
'age': 30,
'city': 'New York'
}
with open('output.yaml', 'w') as file:
yaml.dump(data, file)
This code saves the dictionary data
into a file called output.yaml
. The resulting YAML file would look like this:
age: 30
city: New York
name: John Doe
Handling Japanese Characters
When dealing with Japanese characters in YAML, it is important to specify the allow_unicode=True
option to prevent character encoding issues. This ensures that Japanese characters are correctly displayed in the YAML file.
yaml.dump(data, file, allow_unicode=True)
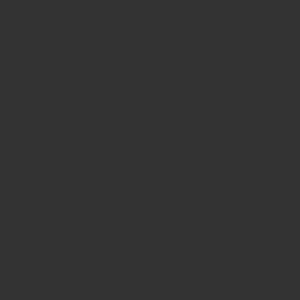
3. Advanced YAML Operations
Creating Custom Tags
In addition to basic data types (lists, dictionaries, etc.), YAML also allows you to serialize and deserialize Python objects. This is done using custom tags. Below is an example of saving a Python class in YAML format.
import yaml
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def person_representer(dumper, data):
return dumper.represent_mapping('!Person', {'name': data.name, 'age': data.age})
def person_constructor(loader, node):
values = loader.construct_mapping(node)
return Person(values['name'], values['age'])
yaml.add_representer(Person, person_representer)
yaml.add_constructor('!Person', person_constructor)
# Convert the object to YAML and save it
person = Person('Alice', 25)
with open('person.yaml', 'w') as file:
yaml.dump(person, file)
# Reconstruct the object from the YAML file
with open('person.yaml', 'r') as file:
loaded_person = yaml.load(file, Loader=yaml.FullLoader)
This way, you can save Python objects in a custom YAML format and later reuse them.
Preserving Order
By default, PyYAML does not preserve the order of dictionaries. If order is important, it is recommended to use ruamel.yaml
. ruamel.yaml
maintains the order of dictionary keys, making it useful when working with configuration files where the order matters.
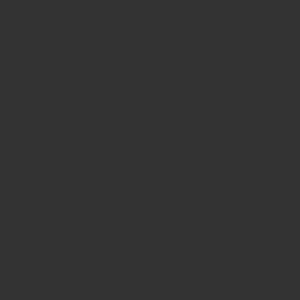
4. YAML Use Cases: Configuration File Management
Convenience of YAML as a Configuration File
YAML is widely used as a configuration file format. In particular, it is an ideal choice for managing configuration data in Python applications. This is because YAML is human-readable and visually easy to understand due to its hierarchical structure. For example, it is well-suited for managing complex configurations, such as database connection information or application logging settings.
database:
host: localhost
port: 3306
username: user
password: pass
logging:
level: DEBUG
file: /var/log/app.log
As shown above, YAML allows for simple, concise descriptions of multiple configurations while maintaining clarity.
Real-World Examples of YAML in Projects
YAML is used in a wide range of projects, including Python frameworks such as Django and Flask, CI tools like CircleCI, and container orchestration tools like Kubernetes. In these projects, YAML is primarily used for configuration management and defining environment variables.
Example of YAML in Django:
In a Django project, YAML can be used to load external configuration files, simplifying deployment and environment setup. By using YAML as a configuration file, different settings for development and production environments can be managed flexibly.
import yaml
with open('config.yaml', 'r') as file:
config = yaml.safe_load(file)
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.mysql',
'NAME': config['database']['name'],
'USER': config['database']['username'],
'PASSWORD': config['database']['password'],
'HOST': config['database']['host'],
'PORT': config['database']['port'],
}
}
Comparison with JSON and XML
YAML stands out for its ease of use as a configuration file format compared to JSON and XML. JSON uses curly braces and commas to separate elements, making long files hard to read. XML requires start and end tags, which often leads to redundancy. On the other hand, YAML uses indentation to represent hierarchical structures, making it intuitive to understand the contents of configuration files.
Comparison of JSON and YAML:
{
"database": {
"host": "localhost",
"port": 3306,
"username": "user",
"password": "pass"
},
"logging": {
"level": "DEBUG",
"file": "/var/log/app.log"
}
}
database:
host: localhost
port: 3306
username: user
password: pass
logging:
level: DEBUG
file: /var/log/app.log
As seen above, YAML is simpler and more readable than JSON.
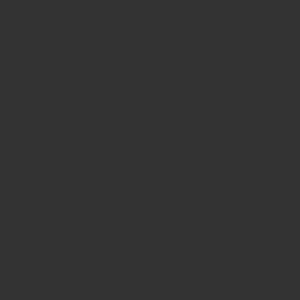
5. Troubleshooting and Error Handling
Common Errors and How to Address Them
One of the most common errors when working with YAML files is encountering issues like “file not found” or “invalid file format.” These errors can be prevented by implementing proper error handling.
For example, when a parsing error occurs in a YAML file, you can catch the exception using yaml.YAMLError
. Additionally, if the file does not exist, you can handle it using FileNotFoundError
and display an appropriate message to the user.
import yaml
def load_yaml(file_path):
try:
with open(file_path, 'r') as file:
data = yaml.safe_load(file)
except FileNotFoundError:
print(f"Error: The file {file_path} does not exist.")
return None
except yaml.YAMLError as e:
print(f"Error: Failed to parse YAML file. {e}")
return None
return data
config = load_yaml('config.yaml')
if config:
print(config)
Best Practices for Error Handling
- File Existence Check: Always check if the file exists, and display an error message if it does not.
- Parsing Error Handling: Catch YAML syntax errors and provide detailed error messages.
- Log Output: When an issue arises, log the error message to a log file to allow for troubleshooting later.
6. Conclusion
YAML is a data serialization format that excels in simplicity and human readability. Reading and writing YAML in Python is straightforward, and it offers many advantages for managing configuration files. Advanced operations, such as creating custom tags, serializing classes, and preserving order, provide even greater flexibility and power in application configuration management.
YAML is widely used not only for configuration file management but also as a format for data storage, and its usage is expected to expand across various projects in the future.