- 1 1. Introduction
- 2 2. Generator Functions and the Basics of yield
- 3 3. Relationship Between Generators and Iterators
- 4 4. Advantages of Using yield and Practical Examples
- 5 5. Handling Sub-Generators with yield from
- 6 6. Applications of Generator Functions and Response Patterns
- 7 7. Conclusion and Next Steps for Learning
1. Introduction
Python is loved by many developers due to its simple syntax and powerful features. Among these features, the yield
keyword is particularly important for optimizing memory efficiency and performance. By using yield
, you can pause and resume iteration while processing data, making it especially useful for handling large datasets or streams of data.
This article will guide you through the basic usage of Python’s yield
keyword, as well as its more advanced applications. Whether you are a beginner or an intermediate programmer, you will find useful information to enhance your programming skills. Please read on until the end.
2. Generator Functions and the Basics of yield
2.1 What is yield
?
yield
is a keyword used within generator functions that temporarily returns a value and pauses the function’s execution. When the function is called again, the yield
will resume execution from where it left off. This feature allows you to process large datasets incrementally, rather than processing them all at once.
def count_up_to(max_value):
count = 1
while count <= max_value:
yield count
count += 1
This function counts up to a specified maximum value and returns one value each time it is called.
2.2 Difference Between return
and yield
While return
ends the execution of a function, yield
only pauses it and allows the function to resume on the next call. This allows you to retrieve values as needed, without loading all the data into memory at once.
def simple_return():
return [1, 2, 3]
This return
version returns the entire list at once, which can increase memory usage.
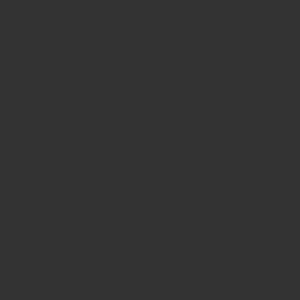
3. Relationship Between Generators and Iterators
3.1 Basics of Iterators
An iterator is an object that returns data one element at a time and implements the __iter__
and __next__
methods. This allows data to be processed sequentially in loops and other constructs. A generator is a type of iterator that can be created easily using yield
.
def custom_generator(start, end):
while start < end:
yield start
start += 1
By using yield
, you can avoid manually implementing an iterator, making data processing more concise.
3.2 Differences Between Iterators and Generators
Generators automatically create iterators by using yield
. In contrast, traditional iterators require explicit implementation of the __iter__
and __next__
methods. This makes generators more concise and easier to maintain.
4. Advantages of Using yield
and Practical Examples
4.1 Improved Memory Efficiency
One of the biggest advantages of using yield
is its improvement of memory efficiency. In a regular function, all the data must be returned at once, but with yield
, data is returned one at a time, which reduces memory usage. This is especially useful when dealing with large datasets or infinite sequences.
For example, yield
is effective when processing large datasets like the following:
def large_data_generator(data):
for item in data:
yield item
This function does not process all the data at once, allowing you to retrieve data as needed and improving performance.
4.2 Practical Scenarios
- Log file processing: When processing log files line by line, using
yield
allows you to handle data efficiently without loading the entire file into memory. - Web scraping: With
yield
, you can process scraped data one item at a time, which is useful for handling large-scale data collection.
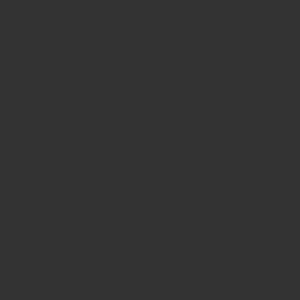
5. Handling Sub-Generators with yield from
5.1 What is yield from
?
yield from
is used to return values directly from an existing generator or iterator. This allows you to combine multiple generators into a single, efficient generator, improving code readability.
def sub_generator():
yield 1
yield 2
yield 3
def main_generator():
yield from sub_generator()
yield 4
In this example, main_generator
returns values from the sub_generator
and then yields 4
as well.
5.2 Practical Example
For instance, when processing data from multiple sources, you can combine the generators for each source and handle the data efficiently. This increases both the flexibility of data processing and the simplicity of your code.
6. Applications of Generator Functions and Response Patterns
6.1 What are Response Patterns?
Generator functions can implement “response patterns” that change their behavior based on external data input. By using yield
, generators can not only return data but also receive values from outside, allowing for two-way communication.
def responder():
response = None
while True:
query = yield response
if query == "Hello":
response = "Hi!"
else:
response = "I don't understand."
6.2 Practical Examples
- Chatbots: Generator functions can be used to implement chatbots that respond to user input.
- State machines: A state machine, which changes behavior based on its state, can be easily handled with
yield
.
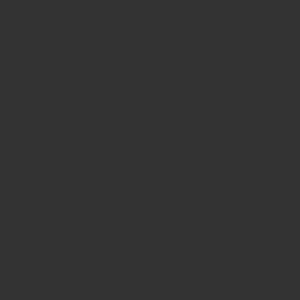
7. Conclusion and Next Steps for Learning
In this article, we have explored the basics and advanced uses of Python’s yield
. yield
is a powerful tool for optimizing memory efficiency and performance, especially useful for handling large datasets and responsive programming.
As your next step, you can further expand your Python programming skills by learning about yield from
and asynchronous programming (async
/await
). By studying the official documentation and working on practical projects, you will deepen your understanding and continue to enhance your Python abilities.