1. What is Python Enum?
Python’s Enum is a class used to implement enumerations, grouping related constants together. Unlike regular data types, using Enum ensures code safety and consistency. It is useful for defining a fixed set of values, such as colors, directions, or states.
1.1 Benefits of Using Enum
- Improved Safety: Enum prevents the use of values outside the defined range, reducing unexpected errors.
- Enhanced Readability: Assigning meaningful names to constants makes the code’s intent clearer and easier to understand.
2. Basic Usage of Enum
To use Enum, first import the enum
module and define a new Enum class by inheriting from the Enum class.
from enum import Enum
class Color(Enum):
RED = 1
BLUE = 2
GREEN = 3
In this example, the Color
class contains three members: RED
, BLUE
, and GREEN
.
2.1 Accessing Enum Members
You can access Enum members using the class name and member name. Additionally, Enums allow retrieval by name or value.
favorite_color = Color.RED
print(favorite_color) # Output: Color.RED
# Retrieve by name
color_by_name = Color['RED']
# Retrieve by value
color_by_value = Color(1)
This makes accessing Enum members straightforward.
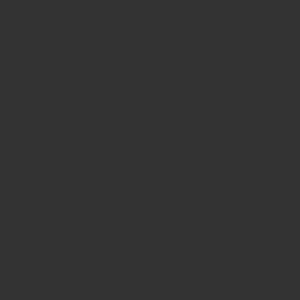
3. Why Use Python Enum?
There are several benefits to using Python Enum.
3.1 Improving Readability
Using Enum makes the code’s intent clearer than using raw numbers or strings. For example, when representing directions, defining meaningful names using Enum improves readability compared to using simple numeric values.
# Without Enum
direction = 1 # It's unclear what this value represents
# Using Enum
class Direction(Enum):
NORTH = 1
SOUTH = 2
EAST = 3
WEST = 4
direction = Direction.NORTH # The intent is clear
3.2 Simplifying Code
With Enum, related constants can be managed collectively, reducing redundancy and improving efficiency. This also eliminates the need to repeatedly define constants.
class Permission(Enum):
READ = 'read'
WRITE = 'write'
EXECUTE = 'execute'
3.3 Preventing Input Errors
Enum restricts values to predefined options, preventing unexpected inputs. For instance, when defining weekdays as an Enum, invalid values cannot be used.
class Weekday(Enum):
MONDAY = 1
TUESDAY = 2
WEDNESDAY = 3
# Using an invalid value will raise an error
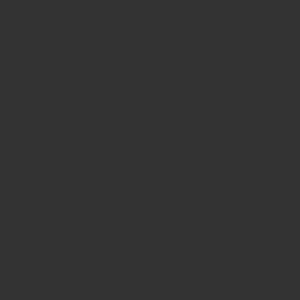
4. Advanced Uses of Enum
Beyond basic usage, Enum can be applied in more advanced ways.
4.1 IntEnum
IntEnum
is a subclass of Enum that treats members as integer values, allowing direct comparison between Enum members and integers.
from enum import IntEnum
class Status(IntEnum):
SUCCESS = 1
FAILURE = 2
print(Status.SUCCESS == 1) # Output: True
4.2 Flag and IntFlag
Flag
and IntFlag
allow combining multiple flags using bitwise operations. This is useful for representing file permissions, for example.
from enum import IntFlag
class Permission(IntFlag):
READ = 1
WRITE = 2
EXECUTE = 4
permissions = Permission.READ | Permission.WRITE
print(permissions) # Output: Permission.READ|WRITE
Using Flag
and IntFlag
makes it easy to manage multiple combined values efficiently.
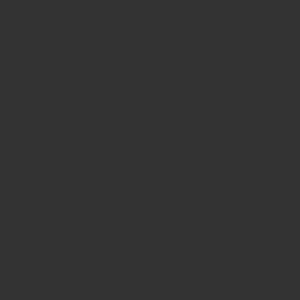
5. Practical Examples of Enum
Enums can be applied in various scenarios. Below are some practical examples.
5.1 Enum for Color Models
When representing RGB colors, Enum can be used to define colors along with their corresponding values.
class RGBColor(Enum):
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
5.2 Managing System States
Enum can be used to manage system or task states. For example, defining task progress using Enum makes the code more understandable.
class TaskStatus(Enum):
PENDING = 'Pending'
IN_PROGRESS = 'In Progress'
COMPLETED = 'Completed'
5.3 Managing Configuration Options
Using Enum for configuration options makes it easier to manage and update options.
class ConfigOption(Enum):
DEBUG = 'debug'
PRODUCTION = 'production'
TESTING = 'testing'
6. Conclusion
Python Enum is a powerful tool for improving code readability, ensuring consistency, and managing related constants efficiently. By organizing constants with Enum, you can reduce redundancy, prevent input errors, and create maintainable, structured code. From basic usage to advanced applications, leveraging Enum enables you to write robust and maintainable programs.