1. What is Python’s append
Method?
The append
method is a fundamental and essential function for list operations in Python. It allows you to add a new element to the end of a list. This feature is extremely useful for dynamically building lists and collecting data.
1.1 Basic Usage
Using append
is very straightforward. You call the append()
method on a list and pass the element you want to add as an argument.
fruits = ['apple', 'banana', 'cherry']
fruits.append('orange')
print(fruits)
When you run this code, ‘orange’ is added to the end of the list, and the output will be:
['apple', 'banana', 'cherry', 'orange']
2. How to Use append
and Important Considerations
While the append
method is simple to use, there are some key points to keep in mind. Most importantly, append
adds a single element to the end of the list—it does not merge entire lists.
2.1 Be Careful When Adding an Entire List
If you use the append
method to add a list, the new list will be added as a single element within the existing list. To add each element separately, use the extend
method instead.
numbers = [1, 2, 3]
numbers.append([4, 5])
print(numbers)
The output will be:
[1, 2, 3, [4, 5]]
As you can see, [4, 5]
has been added as a single element inside the list.
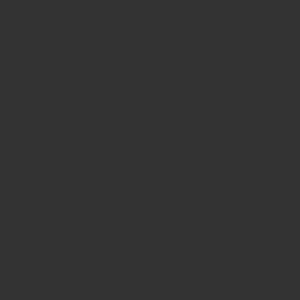
3. Comparison of append
with Other Methods
There are multiple ways to add elements to a list in Python, including append
, extend
, and insert
. Understanding the differences between these methods will help you perform list operations more efficiently.
3.1 The extend
Method
The extend
method is used to add each element from another list or tuple individually to the target list.
numbers = [1, 2, 3]
numbers.extend([4, 5])
print(numbers)
The output will be:
[1, 2, 3, 4, 5]
Since extend
adds elements individually, the list is merged directly without being nested.
3.2 The insert
Method
The insert
method is useful when you need to add an element at a specific position in the list. Unlike append
, which only adds elements at the end, insert
lets you specify the exact index where the new element should go.
numbers = [1, 2, 3]
numbers.insert(1, 'new')
print(numbers)
The output will be:
[1, 'new', 2, 3]
4. Practical Examples of append
The append
method is particularly useful when combined with loops to dynamically build lists. It is often used for collecting user inputs or aggregating data.
4.1 Using append
with Loops
By combining loops with append
, you can add multiple elements sequentially to a list.
numbers = []
for i in range(5):
numbers.append(i)
print(numbers)
The output will be:
[0, 1, 2, 3, 4]
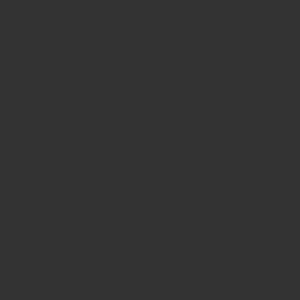
5. Internal Mechanics and Performance of append
The append
method adds elements to the end of a list with an average time complexity of O(1), making it highly efficient. However, since Python lists are internally implemented as dynamic arrays, when the list size exceeds a certain threshold, memory reallocation may be required. This can occasionally lead to performance degradation when performing a large number of append
operations.
5.1 Performance Optimization
When working with large datasets, a high number of append
operations can occur. To optimize performance, consider estimating the initial list size in advance or using an alternative data structure such as collections.deque
. The deque
structure allows efficient appending and removal from both ends, making it ideal for implementing queues or stacks.
6. Alternatives to append
and Best Practices
While append
is the best option for adding elements to the end of a list, it may not always be the optimal choice in every situation. It is important to consider other options when inserting elements at specific positions or when using alternative data structures.
6.1 Utilizing Other Data Structures
If you need to add elements to the beginning of a list, collections.deque
is a more efficient choice. Additionally, if you are performing set operations, using set
instead of a list can lead to better performance. Choosing the appropriate data structure based on the characteristics of your data can significantly improve efficiency.
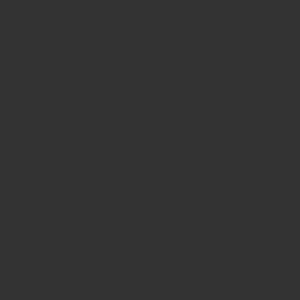
7. Summary
The append
method is a simple yet powerful tool for manipulating lists in Python. By understanding how it works, its internal mechanics, and how it compares to other methods and data structures, you can write more efficient and readable code. From a performance perspective, knowing when to use append
and when to opt for alternative methods is crucial.
This article has provided an in-depth explanation of the append
method, covering its basic usage, internal workings, and performance considerations. By incorporating visual elements and detailed information about data structure choices, readers can gain practical skills for efficient list operations.