1. Basics of Python’s round()
Function
Overview of the round()
Function
The round()
function in Python is a fundamental function used for rounding numbers. It is mainly used to round a number to a specified number of decimal places, allowing for concise adjustment of data display and calculation results.
Basic Syntax
round(number[, ndigits])
- number: The number to be rounded.
- ndigits: The number of decimal places (optional). If omitted, the number is rounded to an integer.
Usage Examples
print(round(3.14159)) # Output: 3
print(round(3.14159, 2)) # Output: 3.14
print(round(1.5)) # Output: 2
As shown above, the round()
function rounds numbers to the specified number of decimal places. If ndigits
is not specified, it rounds to the nearest integer.
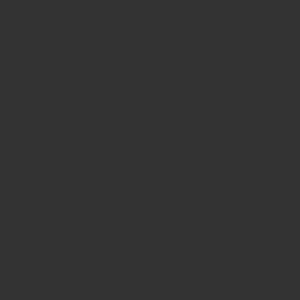
2. Rounding Methods in Python: “Banker’s Rounding”
What Is Banker’s Rounding?
The round()
function in Python uses a method called “Banker’s Rounding.” This technique rounds numbers with a decimal value of 0.5 to the nearest even number. For example, 2.5
is rounded to 2
, while 3.5
is rounded to 4
. This method is commonly used in statistical data and financial calculations to prevent data bias.
Examples of Banker’s Rounding
print(round(2.5)) # Output: 2
print(round(3.5)) # Output: 4
As demonstrated above, when the decimal part is exactly 0.5, the number is rounded to the nearest even integer, which helps prevent cumulative rounding errors.
Advantages of Banker’s Rounding
This method reduces cumulative errors that may arise from always rounding up or down. It is especially useful when processing large datasets, helping to maintain overall balance in numerical calculations.
3. Issues with Floating-Point Numbers
Internal Representation and Errors of Floating-Point Numbers
In Python, floating-point numbers are represented in binary. This can lead to cases where decimal values cannot be represented precisely, sometimes resulting in unexpected rounding outcomes. If a number cannot be accurately represented internally, the result of the round()
function may not match expectations.
Example of Floating-Point Issues
n = 3.15
print(round(n, 1)) # Output: 3.1 (Expected: 3.2)
In this example, 3.15
is internally represented as 3.149999...
, causing the rounding function to produce an unexpected result. This issue arises due to the limitations of floating-point arithmetic.
Why Floating-Point Errors Occur
Since floating-point numbers are stored in binary, certain decimal values like 1.15 or 3.15 cannot be precisely represented. As a result, rounding errors may occur. To mitigate these errors, it is recommended to use high-precision numerical handling, such as the decimal
module, which will be explained next.
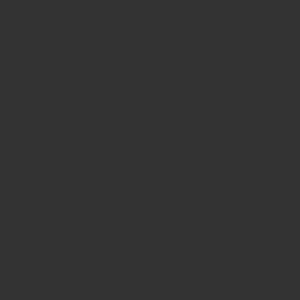
4. Ensuring Precision with the decimal
Module
Overview of the decimal
Module
The decimal
module helps avoid floating-point errors and enables high-precision numerical calculations. It is particularly useful in financial calculations and scientific computations where accuracy is critical.
Usage Example
from decimal import Decimal, ROUND_HALF_UP
n = Decimal('3.15')
print(n.quantize(Decimal('0.1'), rounding=ROUND_HALF_UP)) # Output: 3.2
The Decimal
class eliminates floating-point errors, allowing for highly accurate calculations. The ROUND_HALF_UP
mode performs standard rounding, similar to traditional rounding rules.
Other Rounding Modes
The decimal
module offers various rounding modes, including:
- ROUND_DOWN: Always rounds down.
- ROUND_CEILING: Rounds up for positive numbers and down for negative numbers.
- ROUND_FLOOR: Always rounds toward negative infinity.
These modes provide flexibility for different use cases and allow for precise control over rounding behavior.
5. Other Rounding Methods: math.floor()
and math.ceil()
math.floor()
: Rounding Down
The math.floor()
function rounds down a number to the nearest integer. If the number is negative, it rounds toward negative infinity.
import math
print(math.floor(3.9)) # Output: 3
math.ceil()
: Rounding Up
On the other hand, the math.ceil()
function always rounds a number up to the nearest integer.
import math
print(math.ceil(3.1)) # Output: 4
Difference from round()
Unlike round()
, which rounds to the nearest integer, math.floor()
and math.ceil()
always round in one direction. These functions are particularly useful when predictable rounding behavior is required, even for negative numbers.
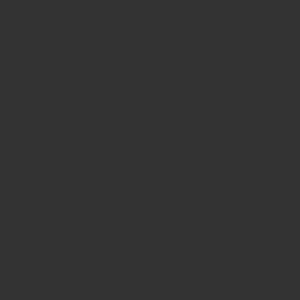
6. Practical Applications
Applying Rounding in Currency Calculations
In currency calculations, precise rounding is essential. Using the decimal
module helps ensure accurate pricing and discount calculations.
from decimal import Decimal, ROUND_HALF_UP
price = Decimal('19.995')
print(price.quantize(Decimal('0.01'), rounding=ROUND_HALF_UP)) # Output: 20.00
Using Rounding in Scientific Calculations
In scientific computations, floating-point errors can lead to inaccurate results. The decimal
module ensures precise calculations, making it useful for handling measurement data and experimental results.
Applying Rounding in Data Analysis
In data analysis, proper rounding helps prevent cumulative errors. When processing statistical or big data, selecting the appropriate rounding method—whether decimal
or round()
—ensures accurate results.
7. Summary
The round()
function in Python is a convenient tool for rounding decimal values, but it is essential to be aware of floating-point precision issues. For financial calculations or cases requiring high accuracy, the decimal
module is recommended. Additionally, understanding alternative rounding methods such as math.floor()
and math.ceil()
allows for greater flexibility in numerical processing.