- 1 1. Overview and Importance of Python Paths
- 2 2. Basic Path Operations: The os.path Module
- 3 3. Advanced Path Operations: The pathlib Module
- 4 4. Utilizing the PYTHONPATH Environment Variable
- 5 5. Choosing Between os.path and pathlib
- 6 6. Frequently Asked Questions (FAQs)
- 6.1 1. How do I get the current working directory in Python?
- 6.2 2. How do I create a directory if it doesn’t exist?
- 6.3 3. What is the difference between absolute and relative paths?
- 6.4 4. Can I use os.path and pathlib together?
- 6.5 5. Why should I use os.path.join() or pathlib’s / operator instead of the + operator?
1. Overview and Importance of Python Paths
Basics of File Path Management in Python
In Python, a “path” is a way to specify the location of files and folders, playing a crucial role in the computer’s file system. For example, when opening a file in a specific directory or manipulating files in a program, incorrect path settings can lead to errors. Understanding how to handle paths is one of the fundamental skills in programming.
Python provides multiple modules for handling file paths, with the most notable being the os.path
module and the pathlib
module. By using these modules correctly, you can efficiently manage files and ensure compatibility across different operating systems.
Absolute Paths vs. Relative Paths
File paths are generally classified into two types: “absolute paths” and “relative paths.”
- Absolute paths specify the full path from the root directory, allowing access to a file or folder regardless of the current working directory. For example, on Windows, an absolute path might look like
C:\Users\YourName\Documents\file.txt
. - Relative paths specify the location of a file relative to the current working directory. For instance, if your current directory is
C:\Users\YourName
, you can access the same file usingDocuments\file.txt
as a relative path.
Why Path Management is Important in Python
When working with files in Python, handling file paths correctly while considering platform differences is crucial. For example, Windows uses a backslash (\
) as the path separator, whereas Linux and macOS use a forward slash (/
). The os.path
and pathlib
modules help manage these differences, allowing you to write cross-platform scripts without worrying about path separators.
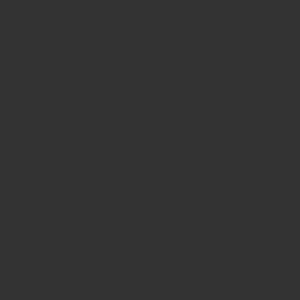
2. Basic Path Operations: The os.path Module
What is the os.path Module?
The os.path
module is part of Python’s standard library and provides convenient functions for handling file and directory paths. It includes basic tools for checking file existence, joining paths, retrieving file names, and more. Additionally, it automatically adapts to different operating systems (Windows, Linux, macOS), making cross-platform development easier.
Key Functions
Checking File or Directory Existence with os.path.exists()
The os.path.exists()
function checks whether a specified path exists. It returns True
if the file or directory exists and False
otherwise. Here’s an example:
import os
path = "/path/to/file.txt"
if os.path.exists(path):
print("The file exists.")
else:
print("The file does not exist.")
Joining Paths with os.path.join()
The os.path.join()
function properly joins multiple path components while considering platform-specific path separators. This eliminates the need for manually concatenating strings. Here’s an example:
import os
dir_path = "/path/to/directory"
file_name = "file.txt"
full_path = os.path.join(dir_path, file_name)
print(full_path) # /path/to/directory/file.txt
Retrieving File Names and Directory Names with os.path.basename() and os.path.dirname()
The os.path.basename()
function extracts the file name from a given path, while os.path.dirname()
extracts the directory name. Here’s how to use them:
import os
path = "/path/to/directory/file.txt"
file_name = os.path.basename(path)
dir_name = os.path.dirname(path)
print(file_name) # file.txt
print(dir_name) # /path/to/directory
Examples of Using the os.path Module
Below is an example demonstrating how to use the os.path
module to check file existence, join paths, and retrieve file and directory names.
import os
# Joining paths
base_dir = "/user/local"
file_name = "example.txt"
full_path = os.path.join(base_dir, file_name)
# Checking if the file exists
if os.path.exists(full_path):
print(f"{full_path} exists.")
else:
print(f"{full_path} does not exist.")
# Extracting file name and directory name
print("File name:", os.path.basename(full_path))
print("Directory name:", os.path.dirname(full_path))
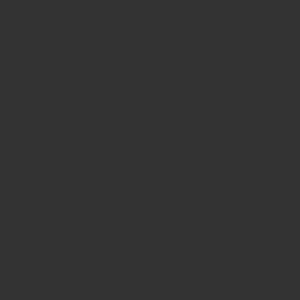
3. Advanced Path Operations: The pathlib Module
Introduction to the pathlib Module
The pathlib
module was introduced in Python 3.4 as an object-oriented approach to handling file system paths. Traditionally, os.path
treated paths as simple strings, requiring multiple functions for path manipulations. In contrast, pathlib
treats paths as objects, making path operations more intuitive and readable.
Basic Usage of pathlib
Creating and Joining Paths
With pathlib
, paths are represented as Path
objects, making them easier to manage. Creating a path is straightforward:
from pathlib import Path
# Creating a path
path = Path("/user/local/example.txt")
print(path)
To join paths, you can use the /
operator, which is a more readable alternative to os.path.join()
:
from pathlib import Path
# Joining paths
base_dir = Path("/user/local")
file_name = "example.txt"
full_path = base_dir / file_name
print(full_path) # /user/local/example.txt
Checking File or Directory Existence
With pathlib
, you can check if a file or directory exists using the exists()
method. To differentiate between files and directories, you can use is_file()
and is_dir()
:
from pathlib import Path
path = Path("/user/local/example.txt")
if path.exists():
print("The file or directory exists.")
if path.is_file():
print("This is a file.")
if path.is_dir():
print("This is a directory.")
Working with Absolute and Relative Paths
The resolve()
method converts a relative path to an absolute path:
from pathlib import Path
relative_path = Path("example.txt")
absolute_path = relative_path.resolve()
print(absolute_path) # /full/path/to/example.txt
To convert an absolute path to a relative path, use relative_to()
:
from pathlib import Path
absolute_path = Path("/user/local/example.txt")
relative_path = absolute_path.relative_to("/user")
print(relative_path) # local/example.txt
Advantages of Using pathlib
The biggest advantage of pathlib
is its object-oriented design, making path operations more intuitive and reducing the need to memorize multiple functions. Compared to os.path
, pathlib
provides better cross-platform support, ensuring compatibility across different operating systems while maintaining clean and concise code.
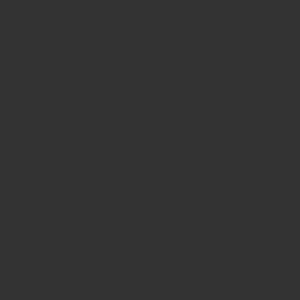
4. Utilizing the PYTHONPATH Environment Variable
What is PYTHONPATH?
PYTHONPATH
is an environment variable that tells Python where to look for modules and packages. By default, Python searches for modules based on sys.path
, which includes the standard library and installed packages. However, setting PYTHONPATH
allows you to prioritize specific directories for module lookup, making it useful for custom module organization and project-specific dependencies.
How to Set PYTHONPATH
Setting PYTHONPATH Temporarily via Command Line
You can temporarily set PYTHONPATH
in the command line before running a Python script:
- For Linux/macOS:
export PYTHONPATH=/path/to/directory:$PYTHONPATH
python script.py
- For Windows:
set PYTHONPATH=C:\path\to\directory;%PYTHONPATH%
python script.py
Since these settings only last for the duration of the terminal session, they are ideal for temporary use.
Setting PYTHONPATH Permanently
To make PYTHONPATH
permanent, you need to modify shell configuration files or system environment variables:
- For Linux/macOS: Add the following line to your
.bashrc
or.zshrc
file:
export PYTHONPATH=/path/to/directory:$PYTHONPATH
- For Windows: Navigate to “System Properties” → “Environment Variables” → “User Environment Variables,” then add a new variable named
PYTHONPATH
with the desired directory path.
This ensures that PYTHONPATH
is set every time a new terminal session starts.
Practical Use Cases of PYTHONPATH
Setting PYTHONPATH
is useful when working on projects with multiple directories containing modules. Consider the following project structure:
/my_project/
│
├── /src/
│ └── my_module.py
│
└── /lib/
└── my_library.py
To make both src
and lib
accessible in Python, set PYTHONPATH
as follows:
export PYTHONPATH=/my_project/src:/my_project/lib
This allows you to import the modules directly in your scripts:
from my_module import my_function
from my_library import my_library_function
Best Practices and Considerations
When setting PYTHONPATH
, be aware of potential conflicts with existing paths. To check the current value of PYTHONPATH
, use:
echo $PYTHONPATH
While PYTHONPATH
is useful for development, it is recommended to use virtual environments (e.g., Virtualenv, venv
) for managing dependencies in production. This avoids conflicts between different projects.
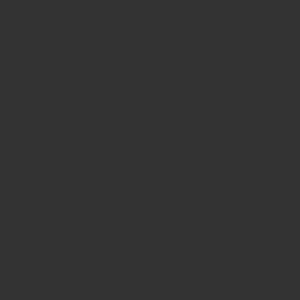
5. Choosing Between os.path and pathlib
Differences Between os.path and pathlib
Python provides two primary modules for handling file paths: os.path
and pathlib
. Each has its own advantages, and choosing the right one depends on the use case.
Features of os.path
os.path
is a traditional module that has been available since early versions of Python. It treats paths as strings and provides function-based operations for handling them. Key features include:
- Lightweight and simple:
os.path
is easy to use for basic path manipulations. - Cross-platform compatibility: Works on Windows, Linux, and macOS.
- String-based operations: Paths are treated as simple strings, making it easy to manipulate them with standard string operations.
Features of pathlib
pathlib
was introduced in Python 3.4 and provides an object-oriented approach to working with paths. It treats paths as Path
objects, making operations more intuitive. Key advantages include:
- Object-oriented design: Paths are objects, allowing for cleaner and more readable code.
- Intuitive path joining: Uses the
/
operator instead of function calls. - Enhanced functionality: Provides built-in methods for common file operations like checking existence, retrieving parent directories, and resolving absolute paths.
When to Use Each Module
When os.path is Suitable
- For legacy systems or Python 2.x compatibility: Since
pathlib
is not available in Python 2.x, usingos.path
ensures compatibility. - For simple scripts and small projects: If you only need basic path manipulations,
os.path
is lightweight and effective.
When pathlib is Recommended
- For new projects using Python 3.x:
pathlib
is the preferred choice for modern Python development. - For complex path operations: If your project involves handling many file paths across different OS environments,
pathlib
offers a cleaner and more maintainable approach. - For large-scale projects: The object-oriented nature of
pathlib
makes code more readable and easier to maintain.
Comparison Table: os.path vs. pathlib
Feature | os.path | pathlib |
---|---|---|
Data Type | String-based | Path objects |
Introduced in | Early Python versions (including 2.x) | Python 3.4+ |
Operation Style | Function-based | Object-oriented |
Path Joining | os.path.join() | / operator |
Absolute Path Resolution | os.path.abspath() | Path.resolve() |
Recommended Use Cases | Simple scripts, legacy projects | New projects, large-scale applications |
Final Recommendation
If you’re using Python 3.4 or later, it is highly recommended to use pathlib
due to its object-oriented design, which makes path handling more intuitive and maintainable. However, if you’re working on older projects or need compatibility with Python 2.x, os.path
remains a valid choice.
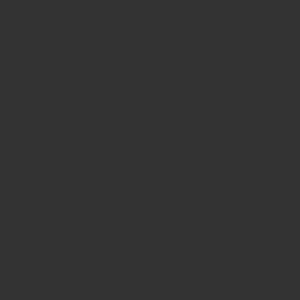
6. Frequently Asked Questions (FAQs)
1. How do I get the current working directory in Python?
You can retrieve the current working directory using either os
or pathlib
:
- Using os:
import os
current_directory = os.getcwd()
print(current_directory)
- Using pathlib:
from pathlib import Path
current_directory = Path.cwd()
print(current_directory)
2. How do I create a directory if it doesn’t exist?
You can create a directory using os.makedirs()
or pathlib.Path.mkdir()
:
- Using os:
import os
dir_path = "/path/to/directory"
if not os.path.exists(dir_path):
os.makedirs(dir_path)
- Using pathlib:
from pathlib import Path
dir_path = Path("/path/to/directory")
dir_path.mkdir(parents=True, exist_ok=True)
3. What is the difference between absolute and relative paths?
- Absolute paths: Start from the root directory (e.g.,
C:\
on Windows,/
on Linux/macOS) and provide the complete path to a file. - Relative paths: Specify a file location relative to the current working directory. For example, if the current directory is
/home/user
, the relative pathdocs/file.txt
refers to/home/user/docs/file.txt
.
4. Can I use os.path and pathlib together?
Yes, both modules can be used in the same project. However, to maintain consistency, it is recommended to stick to one approach. pathlib
is preferred for new projects due to its readability and modern features.
5. Why should I use os.path.join() or pathlib’s / operator instead of the + operator?
Manually concatenating paths with +
can cause issues due to differences in path separators between operating systems (Windows uses \
, while Linux/macOS use /
). Using os.path.join()
or pathlib
ensures correct path formation.
- Incorrect (not recommended):
# Manually joining paths (not recommended)
path = "/user/local" + "/" + "example.txt"
- Correct (recommended):
# Using os.path
import os
path = os.path.join("/user/local", "example.txt")
# Using pathlib
from pathlib import Path
path = Path("/user/local") / "example.txt"
By using the appropriate module, you can ensure compatibility across different operating systems.