1. Basics of the Python `or` Operator
What is the `or` Operator?
The or
operator is one of Python’s logical operators. It returns True
if at least one of the two conditions is True
. It only returns False
when both conditions are False
. This operator is especially useful when combining multiple conditions, enabling concise conditional branching.
Basic Usage
The or
operator is used as follows. When two conditions are connected with or
, the entire expression evaluates to True
if at least one of the conditions is True
.
a = 5
b = 10
if a > 3 or b < 5:
print("At least one condition is true")
else:
print("Both conditions are false")
In this example, a > 3
is True
, while b < 5
is False
. However, since the or
operator is used, the overall evaluation is True
as long as at least one condition is True
.
Characteristics of the `or` Operator
The or
operator has a feature called “short-circuit evaluation.” This means that if the left-side condition is True
, the right-side condition is not evaluated and is skipped. This can be useful for optimizing performance by saving computational resources.
For example, in the following code, since the first condition is True
, the b == 10
condition is not evaluated.
a = 5
b = 10
if a > 3 or b == 10:
print("Evaluation stops here because a is greater than 3")
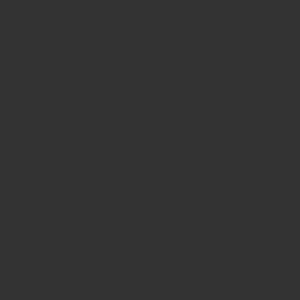
2. Using the `or` Operator in `if` Statements
Using `or` for Multiple Conditions
In Python’s if
statements, the or
operator is very useful for evaluating multiple conditions. For example, if you want to execute a specific action when at least one condition is met, using or
makes the code more concise.
The following example determines actions based on the user’s age using the or
operator.
age = 16
if age < 18 or age > 65:
print("You are eligible for a discount")
else:
print("You are not eligible for a discount")
In this example, users under 18 or over 65 are eligible for a discount. Using or
allows us to combine two conditions simply.
Combining Multiple Conditions with `or`
You can simplify even more complex conditions using the or
operator. In the following example, we use multiple variables to determine if at least one meets a specified condition.
temperature = 35
humidity = 70
if temperature > 30 or humidity > 60:
print("Turn on the air conditioner")
else:
print("No need for air conditioning")
In this case, if the temperature exceeds 30°C or humidity exceeds 60%, the program recommends turning on the air conditioner. The or
operator helps simplify everyday decision-making processes.
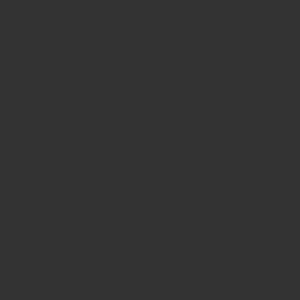
3. Advanced Uses of the `or` Operator
Using the `or` Operator Outside of `if` Statements
The or
operator is not limited to use within if
statements. It can also be useful in various other situations, particularly when setting default values. You can use it to check whether a list, dictionary, or other object is None
or empty and assign a default value if necessary.
def get_list(l=None):
l = l or []
return l
print(get_list()) # Output: []
print(get_list([1, 2, 3])) # Output: [1, 2, 3]
In this example, if the list is None
, an empty list is returned. The or
operator allows for a concise way to handle default values without needing explicit conditionals.
Using Multiple `or` Conditions
In some cases, you might chain three or more conditions using or
. In such cases, the conditions are evaluated from left to right, and the first True
value encountered is returned.
result = None or "default" or "another"
print(result) # Output: default
Here, since None
is treated as False
, the next value, "default"
, is returned. The or
operator is useful for selecting the most appropriate value from multiple conditions.
4. Differences Between `and` and `or`
Fundamental Differences
Both and
and or
are logical operators, but they function very differently. The or
operator returns True
if at least one condition is True
, while the and
operator returns True
only when both conditions are True
.
Let’s look at an example:
a = True
b = False
if a and b:
print("Both conditions are true")
else:
print("At least one condition is false")
In this case, even though a
is True
, b
is False
, so the overall result is False
, and the else
block executes. Compared to the or
operator, the and
operator requires both conditions to be True
for the overall result to be True
.
Using `and` and `or` Together
When combining and
and or
in a single condition, it’s recommended to use parentheses to make the logic clear.
temperature = 25
weather = "rainy"
if (temperature > 20 and temperature < 30) or weather == "rainy":
print("Take an umbrella with you")
else:
print("No umbrella needed")
In this case, the program advises taking an umbrella if the temperature is between 20°C and 30°C or if the weather is rainy. By combining and
and or
, you can construct complex conditions concisely.
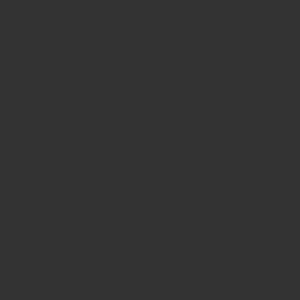
5. Optimizing Performance with the `or` Operator
Performance Benefits of Short-Circuit Evaluation
One of the biggest advantages of the or
operator is its “short-circuit evaluation” property. If the left-hand condition evaluates to True
, the right-hand condition is not evaluated, preventing unnecessary computations and improving performance.
For example, in the following code, can_publish()
is never executed because can_edit()
returns True
:
def can_edit():
return True
def can_publish():
print("This function will not be called")
return True
if can_edit() or can_publish():
print("Editing or publishing is allowed")
Since the left-hand condition can_edit()
returns True
, the or
operator does not evaluate the right-hand condition can_publish()
. This behavior is particularly useful for improving efficiency in large-scale applications.
Practical Use Cases
When handling large datasets or processing multiple conditions efficiently, short-circuit evaluation helps reduce execution time. Using the or
operator strategically allows you to avoid unnecessary operations and write optimized code.
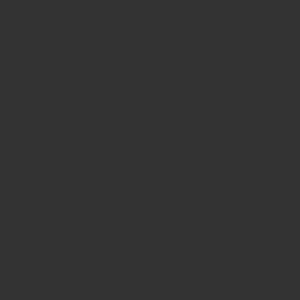
6. Conclusion
Reviewing the `or` Operator
The or
operator plays a crucial role in Python programming, particularly in logical operations. It returns True
if at least one condition is True
, making it especially useful in conditional statements and default value settings. Additionally, since or
supports short-circuit evaluation, it contributes to performance optimization.
Try It in Your Code
Beyond just learning the theory, it’s highly recommended to practice using the or
operator in a Python development environment. Try different use cases, from basic applications to advanced scenarios, and observe how the code behaves. By experimenting with conditions and writing efficient code, you’ll develop a deeper understanding of the or
operator.