1. Introduction
Python is a versatile programming language that provides powerful tools for data processing, machine learning, and web development. Among these, the multiprocessing module is an essential library for implementing parallel processing. In this article, we will provide a detailed explanation of how to use Python’s multiprocessing module, from the basics to advanced techniques, with visual aids and practical strategies to maximize performance.
2. What is multiprocessing?
2.1 The Need for Parallel Processing
By default, Python runs in a single-threaded mode, which can become a bottleneck when handling computationally intensive tasks or large datasets. Utilizing parallel processing allows multiple tasks to run simultaneously, making full use of all CPU cores and reducing processing time. The multiprocessing module bypasses Python’s Global Interpreter Lock (GIL) and enables true parallel processing by utilizing multiple processes.
2.2 Difference from Single-Threading
In a single-threaded setup, a single process executes tasks sequentially, whereas multiprocessing allows multiple processes to run concurrently. This significantly improves performance, particularly for CPU-bound tasks such as large-scale numerical computations and data analysis.
3. Basic Syntax of the multiprocessing Module
3.1 Using the Process Class
The foundation of the multiprocessing
module is the Process class, which makes it easy to create new processes and execute tasks in parallel.
import multiprocessing
def worker_function():
print("A new process has started")
if __name__ == "__main__":
process = multiprocessing.Process(target=worker_function)
process.start()
process.join()
In this example, the worker_function
is executed in a new process. The start()
method launches the process, and the join()
method waits for its completion.
3.2 Passing Arguments to a Process
To pass arguments to a process, use the args
parameter. The following example demonstrates passing an argument to the worker
function.
def worker(number):
print(f'Worker {number} has started')
if __name__ == "__main__":
process = multiprocessing.Process(target=worker, args=(5,))
process.start()
process.join()
This allows dynamic data to be passed into a process, enabling concurrent execution with different inputs.
4. Data Sharing and Synchronization
4.1 Sharing Data Using Shared Memory
When using multiple processes, Value and Array can be used to safely share data between them. These objects enable multiple processes to access shared memory without causing data corruption.
import multiprocessing
def increment_value(shared_value):
with shared_value.get_lock():
shared_value.value += 1
if __name__ == "__main__":
shared_value = multiprocessing.Value('i', 0)
processes = [multiprocessing.Process(target=increment_value, args=(shared_value,)) for _ in range(5)]
for process in processes:
process.start()
for process in processes:
process.join()
print(f'Final value: {shared_value.value}')
In this example, five processes increment a shared integer value. The get_lock()
method prevents race conditions by ensuring safe access to shared memory.
4.2 Preventing Data Races with Locks
When multiple processes access and modify shared data simultaneously, a locking mechanism can prevent race conditions. Using a Lock
object ensures synchronization between processes.
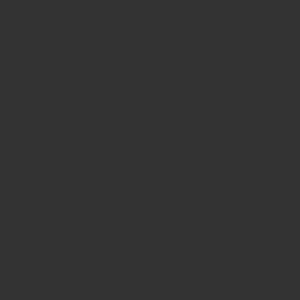
5. Distributing Tasks with Process Pools
5.1 Utilizing the Pool Class
The Pool
class enables the distribution of multiple tasks across multiple processes, making it extremely useful for large-scale data processing and task delegation.
from multiprocessing import Pool
def square(x):
return x * x
if __name__ == "__main__":
with Pool(4) as pool:
results = pool.map(square, range(10))
print(results)
In this example, the square of each number in the list is computed and distributed across four processes in parallel. The map()
function efficiently assigns tasks to processes.
Diagram: Task Distribution with the Pool Class
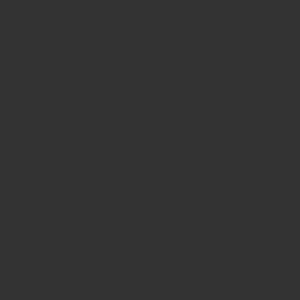
5.2 Advanced Use: Processing Multiple Arguments with starmap
The starmap()
function allows functions with multiple arguments to be processed in parallel. The following example demonstrates how to execute a function with argument pairs.
def multiply(x, y):
return x * y
if __name__ == "__main__":
with Pool(4) as pool:
results = pool.starmap(multiply, [(1, 2), (3, 4), (5, 6), (7, 8)])
print(results)
6. Optimizing CPU Resource Usage
6.1 Optimizing Process Count with cpu_count()
Using Python’s multiprocessing.cpu_count()
function, you can automatically determine the number of physical CPU cores and adjust the number of processes accordingly. This prevents excessive process creation and optimizes overall system performance.
from multiprocessing import Pool, cpu_count
if __name__ == "__main__":
with Pool(cpu_count() - 1) as pool:
results = pool.map(square, range(100))
print(results)
6.2 Efficient Use of System Resources
Instead of using all CPU cores, it is advisable to leave one core free for system tasks. This ensures that parallel processing does not negatively impact other running applications.
7. Real-World Use Cases and Best Practices
7.1 Practical Use Cases
The multiprocessing
module is highly effective in the following scenarios:
- Large-Scale Data Processing: Useful for simultaneously reading and processing multiple files.
- Parallel Training in Machine Learning: Enables simultaneous execution of model training processes, reducing training time.
- Web Crawling: Facilitates efficient data collection by crawling multiple web pages in parallel.
7.2 Best Practices
- Optimal Resource Allocation: Adjust the number of processes based on the number of physical CPU cores.
- Debugging and Logging: Utilize the
logging
module to track process status and handle errors effectively.
import logging
import multiprocessing
def worker_function():
logging.info(f'Process {multiprocessing.current_process().name} has started')
if __name__ == "__main__":
logging.basicConfig(level=logging.INFO)
process = multiprocessing.Process(target=worker_function, name='Worker 1')
process.start()
process.join()
This example uses logging
to record process activity, making it easier to analyze logs later.
- Implementing Error Handling: Since multiple processes run simultaneously, error handling is crucial. Use
try-except
statements to prevent failures in one process from affecting others.
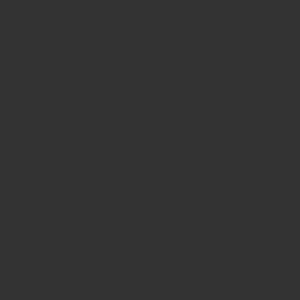
8. Conclusion
In this article, we explored how to efficiently implement parallel processing using Python’s multiprocessing
module. From the basics of the Process class to data sharing, task distribution with process pools, and real-world use cases, we covered practical techniques for leveraging multiprocessing effectively.
By mastering parallel processing, you can significantly enhance performance in projects involving large-scale data processing, machine learning model training, web crawling, and more. The multiprocessing
module is a powerful tool that enables efficient use of system resources and dramatically improves Python’s processing capabilities.
We encourage you to apply these techniques to your development projects and take full advantage of Python’s multiprocessing capabilities.