1. Introduction
Python lists are a highly versatile data structure for handling data. The ability to merge multiple lists is especially useful in data science and web application development. Merging lists is essential for centralizing data management and efficiently processing large datasets. In this article, we will introduce five methods for merging lists in Python, explaining their use cases and performance differences. Whether you’re a beginner or an advanced user, you’ll find this guide valuable.
2. Fundamentals of Merging Lists
2.1 What Are Lists in Python?
Lists in Python are a flexible data type that can store elements of different types. They can contain numbers, strings, and even other lists, and their elements can be accessed in any order. Merging lists is a crucial operation for consolidating multiple datasets into a single list, significantly improving data processing efficiency.
2.2 Purpose and Use Cases of Merging Lists
Merging lists is commonly used in the following scenarios:
- Data Science: Combining multiple datasets for analysis.
- Web Scraping: Aggregating data collected from different web pages into a single list for further processing.
- API Data Integration: Merging data retrieved from multiple APIs for unified processing.
Understanding how to merge lists allows for more flexible data manipulation and improves program performance.
3. Five Methods to Merge Lists
Python offers various methods for merging lists. This section provides a detailed explanation of each method and its characteristics.
3.1 Merging Lists with the “+” Operator
The “+” operator allows you to concatenate multiple lists, creating a new merged list. While this method is simple and easy to understand, it consumes more memory as it generates a new list.
list1 = [1, 2, 3]
list2 = [4, 5, 6]
result = list1 + list2
print(result) # [1, 2, 3, 4, 5, 6]
- Advantages: Easy and straightforward list merging.
- Disadvantages: Creates a new list, consuming more memory for large datasets.
3.2 Merging Lists with the “+=” Operator
The +=
operator updates an existing list by appending another list in place. This method is more memory-efficient since it modifies the original list directly.
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list1 += list2
print(list1) # [1, 2, 3, 4, 5, 6]
- Advantages: More memory-efficient as it modifies the original list directly.
- Disadvantages: Alters the original list, which may not always be desirable.
3.3 Merging Lists with the extend()
Method
The extend()
method adds elements from another list to the original list. It works similarly to +=
but makes the intent clearer, improving code readability.
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list1.extend(list2)
print(list1) # [1, 2, 3, 4, 5, 6]
- Advantages: Similar to
+=
, but improves readability. - Disadvantages: Modifies the original list, making it unsuitable if you need to retain a copy.
3.4 Adding Lists with the append()
Method
The append()
method adds an entire list as a single element at the end of another list. This method is useful when you want to create a nested list.
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list1.append(list2)
print(list1) # [1, 2, 3, [4, 5, 6]]
- Advantages: Useful for creating nested lists.
- Disadvantages: Not suitable for typical list merging.
3.5 Merging String Lists with the join()
Method
The join()
method is useful when merging a list of strings into a single string, such as combining words into a sentence.
words = ['Python', 'is', 'fun']
sentence = ' '.join(words)
print(sentence) # "Python is fun"
- Advantages: Ideal for merging lists of strings.
- Disadvantages: Cannot be used for lists containing non-string elements.
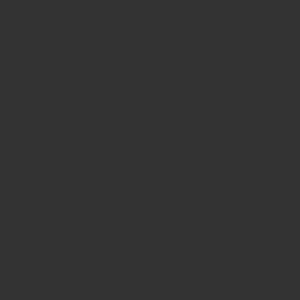
4. Choosing the Best Merging Method for Different Use Cases
The best merging method depends on the use case. For small lists, the “+” operator is simple and effective, while extend()
and +=
are better for handling large datasets efficiently. If you need a nested list, append()
is the right choice, whereas join()
is ideal for merging string lists.
5. Avoiding Errors and Efficient List Merging
One common error when merging lists is a TypeError
. For example, attempting to pass multiple arguments to the append()
method will result in an error.
list1 = [1, 2, 3]
list1.append(4, 5) # TypeError: append() takes exactly one argument (2 given)
To avoid such errors, using extend()
or +=
is a safer approach. Regarding performance, as the dataset size increases, the “+” operator becomes less efficient, so extend()
or +=
should be used for better efficiency.
6. Practical Use Cases: Applying List Merging
6.1 Merging CSV Data
When processing data from multiple CSV files, list merging can be useful. By using extend()
or +=
, you can aggregate rows from different CSV files into a single list for further analysis and processing.
6.2 Aggregating Web Scraping Results
When performing web scraping, data is often stored in lists. Merging lists is useful for combining data collected from multiple pages, enabling efficient data aggregation and processing.
7. Conclusion
There are five main methods for merging lists in Python: the “+” operator, +=
, extend()
, append()
, and join()
. Each method has its own best use case. When handling large datasets, considering memory efficiency and performance is crucial. Using extend()
or +=
allows for efficient merging without creating copies, while join()
is particularly useful for merging lists of strings.
Incorrectly merging lists can lead to errors or unexpected behavior, so understanding and utilizing the right method is essential for effective Python development.