- 1 1. The Convenience of Working with Excel Files in Python
- 2 2. Introduction to Key Libraries for Reading Excel Files in Python
- 3 3. Pandas vs. OpenPyXL: Which One Should You Choose?
- 4 4. Practical Examples: From Reading Excel Files to Data Processing
- 5 5. Best Practices and Considerations When Working with Excel Files
- 6 6. Conclusion: Enhancing Excel Operations with Python
1. The Convenience of Working with Excel Files in Python
1.1 Background
Excel is widely used for data management and business report creation, playing a crucial role as a tool for efficient data processing. However, manually handling data can be time-consuming and prone to errors. By using Python automation scripts to read and process Excel data, you can significantly improve efficiency and accuracy.
1.2 The Strengths of Python
Python is a programming language capable of performing complex tasks with concise code. By leveraging libraries like Pandas and OpenPyXL, you can easily read and edit Excel files. Utilizing Python’s versatility can greatly enhance workflow automation and efficiency.
2. Introduction to Key Libraries for Reading Excel Files in Python
2.1 Reading Excel Files with Pandas
Pandas is a Python library specialized for data analysis and manipulation, making it easy to read Excel files. The read_excel()
function allows you to import Excel data into a DataFrame, facilitating data processing and analysis.
import pandas as pd
# Read an Excel file
df = pd.read_excel('example.xlsx')
print(df)
Handling Multiple Sheets
Pandas can also easily handle Excel files with multiple sheets. Using sheet_name=None
, you can retrieve all sheets as a dictionary.
df_sheets = pd.read_excel('example.xlsx', sheet_name=None)
for sheet_name, df in df_sheets.items():
print(f"Sheet: {sheet_name}")
print(df)
2.2 Reading Excel Files with OpenPyXL
OpenPyXL is a library that supports editing and formatting Excel files, making it ideal when you need to manipulate specific cells or rows directly. Additionally, it preserves Excel layouts and charts, making it suitable for automating business document creation.
from openpyxl import load_workbook
# Load an Excel file
wb = load_workbook('example.xlsx')
ws = wb['Sheet1']
# Get a cell value
cell_value = ws['A1'].value
print(cell_value)
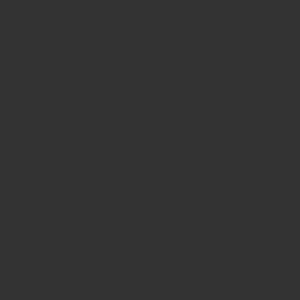
3. Pandas vs. OpenPyXL: Which One Should You Choose?
3.1 Differences in Performance
Pandas is highly efficient for aggregating and filtering large datasets but may consume significant memory when handling large Excel files. On the other hand, OpenPyXL is more memory-efficient and allows for efficient reading of Excel files using the read_only=True
option.
# OpenPyXL read-only mode
wb = load_workbook('large_file.xlsx', read_only=True)
3.2 Features and Versatility
Pandas is ideal for data analysis and statistical processing, offering a convenient way to manipulate data in DataFrame format. Meanwhile, OpenPyXL excels at editing Excel files, preserving VBA scripts, and creating charts, making it perfect for direct Excel file manipulation.
4. Practical Examples: From Reading Excel Files to Data Processing
4.1 Basic Excel File Reading
A simple example of reading an Excel file using Pandas.
df = pd.read_excel('sales_data.xlsx')
print(df)
4.2 Manipulating Specific Sheets and Cells
Using OpenPyXL to retrieve data from specific sheets or cells and write new data.
from openpyxl import load_workbook
wb = load_workbook('sales_data.xlsx')
ws = wb['2023']
print(ws['A1'].value)
# Write new data
ws['B1'] = 'New Data'
wb.save('updated_sales_data.xlsx')
4.3 Filtering and Aggregating Data
Filtering and aggregating data based on specific conditions using Pandas.
filtered_df = df[df['Date'].between('2023-09-01', '2023-09-30')]
total_sales = filtered_df['Sales'].sum()
print(f"Total sales in September: {total_sales}")
5. Best Practices and Considerations When Working with Excel Files
5.1 Implementing Error Handling
When reading Excel files, it is crucial to implement error handling to account for situations where the file does not exist or has an unexpected data format.
try:
df = pd.read_excel('non_existent_file.xlsx')
except FileNotFoundError as e:
print(f"Error: File not found: {e}")
5.2 Considerations for Character Encoding and Formatting
If your Excel file contains non-English characters, setting the correct encoding is essential to prevent text corruption.
df = pd.read_csv('data.csv', encoding='utf-8')
5.3 Efficiently Processing Large Datasets
To handle large datasets efficiently, use the chunksize
option in Pandas or the read_only
mode in OpenPyXL.
# Using Pandas' chunksize option
chunks = pd.read_csv('large_data.csv', chunksize=1000)
for chunk in chunks:
print(chunk)
5.4 Preserving Formatting and Creating Charts with OpenPyXL
OpenPyXL allows you to preserve cell formatting while adding or modifying data. It also supports creating charts in Excel.
from openpyxl.chart import BarChart, Reference
# Create a bar chart
chart = BarChart()
data = Reference(ws, min_col=2, min_row=1, max_col=2, max_row=10)
chart.add_data(data, titles_from_data=True)
ws.add_chart(chart, "E5")
6. Conclusion: Enhancing Excel Operations with Python
Pandas and OpenPyXL are both powerful tools that serve different purposes. Pandas is ideal for data analysis, while OpenPyXL excels at manipulating Excel files directly. By using the right tool for the job, you can significantly improve the efficiency of working with Excel files. Python enables workflow automation and advanced data processing, boosting productivity and reducing manual effort.