1. Introduction
Python is widely used in various programs, and how a program terminates plays a crucial role in application control and resource management. In this article, we will explain the differences and specific usage of the three Python termination methods: exit()
, sys.exit()
, and os._exit()
. Additionally, we will explore best practices for handling exit codes and resource management.
2. Basics of the exit()
Function
2.1 What is exit()
?
exit()
is included in Python’s standard library and provides a simple way to terminate a session in interactive shell mode. Since it is not typically used in scripts, unlike sys.exit()
, it does not handle error exceptions or return an exit code to the system.
exit()
2.2 When to Use exit()
exit()
is useful for terminating an interactive shell but is insufficient for use in scripts. Since it does not return an exit code to the system, it should be limited to debugging or testing environments.
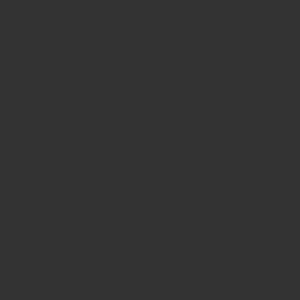
3. Understanding sys.exit()
3.1 What is sys.exit()
?
sys.exit()
is the standard way to terminate a Python program. It allows you to specify an exit code, where 0 indicates a normal exit, and any non-zero value indicates an abnormal termination. The exit code is crucial as it helps external systems and scripts handle the program’s result appropriately.
import sys
sys.exit(0) # Normal exit
sys.exit(1) # Abnormal exit
3.2 SystemExit
Exception and Exception Handling
sys.exit()
raises a SystemExit
exception during execution. This allows for cleanup operations or post-processing before termination by handling the exception. The following example demonstrates catching SystemExit
to display a message before exiting:
import sys
try:
sys.exit("Error message")
except SystemExit as e:
print(f"Program terminated: {e}")
3.3 Cleanup and Resource Management
When using sys.exit()
, ensuring proper cleanup is essential. Open files, network connections, and other resources should be released properly. The finally
block can be used to guarantee resource release.
import sys
try:
# Performing operations on resources
print("Working with files or network resources")
finally:
# Resource cleanup process
print("Releasing resources")
sys.exit(0)
4. How to Use os._exit()
and Important Considerations
4.1 Overview of os._exit()
os._exit()
is a low-level function that forcefully terminates the entire process without performing normal cleanup or exception handling. It is primarily used in multi-process applications where safely terminating child processes is necessary.
import os
os._exit(0)
4.2 When to Use os._exit()
os._exit()
is used when normal termination processing is not required, especially in multi-process environments. For example, it is useful for safely terminating a child process created by fork()
without affecting the parent process.
import os
pid = os.fork()
if pid == 0:
# Child process execution
print("Child process exiting")
os._exit(0)
else:
# Parent process execution
print("Parent process running")
4.3 Risks in Resource Management
Since os._exit()
does not perform cleanup operations, open files and sockets may remain unreleased. It is recommended to manually close necessary resources before calling os._exit()
. Failing to do so can lead to resource leaks and negatively impact system performance.
5. Comparing Real-World Use Cases
5.1 Choosing the Right Termination Method
The appropriate termination method depends on the needs of your application.
exit()
: Used for terminating a Python session in interactive shell mode.sys.exit()
: The most common method for terminating a program, useful when exception handling and cleanup are required.os._exit()
: Used when an immediate process termination is necessary, such as in a multi-process environment where a child process needs to exit without affecting the parent process.
5.2 Example Use Cases and Considerations
# Example usage of sys.exit()
import sys
try:
for i in range(10):
if i == 5:
print(f"Terminating program at iteration {i}")
sys.exit(0)
finally:
print("Releasing resources")
As seen above, sys.exit()
is optimal for scenarios where cleanup and post-exit operations are necessary. In contrast, when using os._exit()
, resources will not be automatically freed, requiring extra caution.
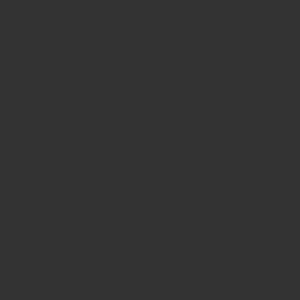
6. Exit Codes and Shell Script Integration
6.1 Using Exit Codes
By specifying exit codes when using sys.exit()
or os._exit()
, external systems and shell scripts can make decisions based on the program’s outcome. Exit codes are useful for detecting failures and coordinating processes between scripts.
python script.py
echo $?
6.2 Example of Integration with a Shell Script
The following shell script handles errors based on the exit code of a Python script:
#!/bin/bash
python script.py
if [ $? -eq 0 ]; then
echo "Exited successfully."
else
echo "An error occurred."
fi
By leveraging exit codes, you can implement reliable error handling, improving the stability and maintainability of your system.
7. Conclusion
In this article, we explored the differences and proper usage of Python’s program termination methods: exit()
, sys.exit()
, and os._exit()
. Each method has its own best use case, and choosing the right one ensures program reliability and efficiency.
In particular, sys.exit()
is highly recommended when exception handling and resource management are necessary. It allows for safe resource release and ensures that logs or final messages are correctly outputted.
Meanwhile, os._exit()
is suited for special cases where normal termination is not feasible. It is useful in multi-process environments or when immediate system shutdown is required.
The choice of termination method depends on the structure and processing needs of your program. However, in most cases, sys.exit()
should be the preferred choice, while os._exit()
should be used only when absolutely necessary. Always design your program to ensure resources are properly released upon termination, enhancing system stability and reliability.
References and Additional Reading
Refer to these resources for detailed information on Python’s termination methods and official recommendations for various usage scenarios.