- 1 1. Introduction
- 2 2. Method 1: A Simple Approach Using the in Operator
- 3 3. Method 2: Finding the Index Using the find() Method
- 4 4. Method 3: Searching for the Last Occurrence Using the rfind() Method
- 5 5. Method 4: Advanced Searching with Regular Expressions (re.search())
- 6 6. Comparison and Best Use Cases for Each Method
- 7 7. Conclusion
1. Introduction
The Importance of String Manipulation in Python
When programming in Python, string manipulation is an essential skill that is frequently required. Checking whether a specific word or phrase is present in a string is useful in various scenarios, such as data processing, text analysis, and web scraping.
In this article, we will introduce four main methods to check if a string contains a specific substring in Python. Understanding these techniques will help you improve program efficiency and avoid errors.
2. Method 1: A Simple Approach Using the in
Operator
What is the in
Operator?
In Python, the simplest way to check if a string contains a specific substring is by using the in
operator.
This method is easy to understand, even for beginners, and provides excellent code readability. The in
operator returns True
if the substring is found and False
if it is not.
Example Usage
text = "Python is a versatile language."
print("versatile" in text) # True
print("java" in text) # False
In the above code, the substring "versatile"
is found within text
, so it returns True
.
On the other hand, since "java"
is not present, it returns False
.
Advantages and Disadvantages of the in
Operator
Advantages
- Short and intuitive code.
- Returns a boolean (
True
orFalse
), making it easy to use in conditional statements.
Disadvantages
- Not suitable for case-sensitive searches or partial matches.
- While useful for simple searches, it does not provide position information or support complex patterns.
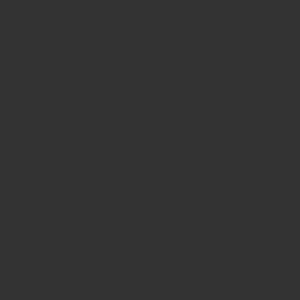
3. Method 2: Finding the Index Using the find()
Method
What is the find()
Method?
The find()
method returns the index of the first occurrence of a substring within a string.
If the substring is found, it returns an index (0 or greater). If it is not found, it returns -1
.
Since it is case-sensitive, you may need to adjust accordingly.
Example Usage
text = "apple, orange, banana"
index = text.find("orange")
print(index) # 7
In this example, the substring "orange"
appears at the 7th position in text
, so 7
is returned.
If you search for "grape"
, it will return -1
because it is not found.
Advanced Use: Ignoring Case Sensitivity
To perform a case-insensitive search, you can use the lower()
method to convert the entire string to lowercase before searching.
text = "Python is Great"
index = text.lower().find("great")
print(index) # 10
Advantages and Disadvantages of the find()
Method
Advantages
- Provides the position of the first occurrence, allowing for position-based operations.
- Simple and easy to use.
Disadvantages
- Case-sensitive, requiring additional processing if case insensitivity is needed.
- Only returns the first occurrence and does not handle multiple matches.
4. Method 3: Searching for the Last Occurrence Using the rfind()
Method
What is the rfind()
Method?
The rfind()
method searches from the right side of a string and returns the index of the last occurrence of a substring.
It operates in the opposite way of the find()
method. If the substring is not found, it returns -1
.
Example Usage
text = "apple, orange, apple, banana"
index = text.rfind("apple")
print(index) # 14
In this example, the last occurrence of "apple"
is found at index 14
, so 14
is returned.
Unlike find()
, which searches from the left, rfind()
searches from the right and returns the last match.
Practical Applications of the rfind()
Method
The rfind()
method is useful when dealing with repeated occurrences of a substring and you need to focus on the last occurrence.
For example, it can be used in log files or long text documents to locate the last instance of an error message or a keyword.
Advantages and Disadvantages of the rfind()
Method
Advantages
- Effective for identifying the last occurrence of a substring.
- Useful for large-scale text analysis and log file processing.
Disadvantages
- Cannot retrieve multiple occurrences at once.
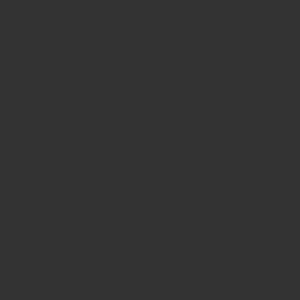
5. Method 4: Advanced Searching with Regular Expressions (re.search()
)
What Are Regular Expressions?
Regular expressions (Regex) are powerful tools for performing pattern matching in strings.
In Python, the re
module allows for advanced searches using regular expressions.
This method is particularly useful for identifying specific patterns and performing flexible partial matches.
Example Usage: re.search()
import re
text = "apple, orange, banana"
match = re.search(r"ora[a-z]*", text)
if match:
print(match.group()) # orange
In this example, we search for a word that starts with "ora"
and continues with lowercase letters.
The regular expression successfully finds and returns "orange"
.
By using regular expressions, more flexible searches become possible.
Advanced Example: Searching for Multiple Patterns
To search for multiple patterns at the same time, you can specify different conditions using the re
module.
For example, this is useful when searching for numbers or special characters in a string.
match = re.search(r"\d+", "apple 123 banana")
if match:
print(match.group()) # 123
Advantages and Disadvantages of Regular Expressions
Advantages
- Allows for flexible and complex pattern matching.
- Ideal for large-scale text analysis and pattern extraction.
Disadvantages
- Regex syntax can be difficult, requiring a learning curve for beginners.
- May be slower compared to simpler string search methods.
6. Comparison and Best Use Cases for Each Method
Comparison Table
Method | Function | Advantages | Disadvantages |
---|---|---|---|
in | Checks for substring existence | Simple and fast | Case-sensitive |
find() | Finds the index of the first occurrence | Provides position information | Only finds the first match |
rfind() | Finds the last occurrence from the right | Retrieves the last match | Only finds the last match |
re.search() | Uses regular expressions | Flexible and supports complex patterns | High learning curve, slower execution |
Recommended Use Cases
- For simple substring searches, use the
in
operator. - To obtain the position of a match, use
find()
orrfind()
. - For complex pattern matching, use regular expressions.
7. Conclusion
Python provides various methods to check if a string contains a specific substring, ranging from simple to advanced techniques.
In this article, we covered the in
operator, find()
, rfind()
, and the re.search()
function using regular expressions.
Each method has its own strengths and weaknesses, so choosing the right one depends on the situation.
- For simple searches, use the
in
operator. - For finding positions of matches, use
find()
orrfind()
. - For complex searches, use regular expressions.
By selecting the appropriate method for your needs, you can efficiently perform string searches in your Python programs.
If you have any questions or thoughts, feel free to share them in the comments section!