- 1 1. The Need for Splitting Strings in Python
- 2 2. Basic String Splitting with Python’s split() Method
- 3 3. Splitting Strings Based on Newline Characters with splitlines()
- 4 4. Splitting Strings Using Regular Expressions with re.split()
- 5 5. Other Useful Methods: partition() and rpartition()
- 6 6. Practical Examples: Applying String Splitting in Python
- 7 7. Summary: Mastering String Splitting Methods in Python
1. The Need for Splitting Strings in Python
1.1. Importance of String Manipulation
String manipulation is crucial in Python. Whether processing everyday text data or performing preprocessing for data analysis, splitting strings is often required. For example, string splitting is essential when parsing CSV files, handling user input, or processing text data obtained through web scraping.
1.2. What You Will Learn in This Article
This article covers a wide range of techniques for splitting strings in Python, from basic methods to more advanced approaches using regular expressions. We will focus on key methods like split()
, splitlines()
, and re.split()
, providing practical code examples to help you understand their usage.
2. Basic String Splitting with Python’s split() Method
2.1. What is the split() Method?
The split()
method is one of the most fundamental string manipulation methods in Python. It splits a string based on a specified delimiter and returns a list. By default, whitespace characters (spaces, tabs, newlines) are used as delimiters.
text = "apple banana cherry"
fruits = text.split()
print(fruits) # Output: ['apple', 'banana', 'cherry']
2.2. Specifying a Delimiter
You can specify a delimiter to split a string. For example, if you want to split a string based on commas or semicolons, you can do the following:
text = "apple,banana,cherry"
fruits = text.split(",")
print(fruits) # Output: ['apple', 'banana', 'cherry']
2.3. Limiting the Number of Splits
The second argument of the split()
method allows you to limit the number of splits, controlling how many times the string is divided.
text = "apple,banana,cherry,orange"
fruits = text.split(",", 2)
print(fruits) # Output: ['apple', 'banana', 'cherry,orange']
This feature is useful when you need to extract only specific portions while preserving the rest of the string.
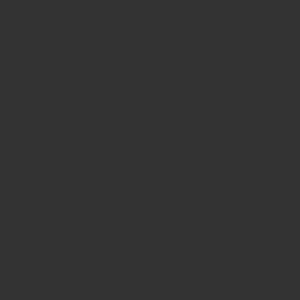
3. Splitting Strings Based on Newline Characters with splitlines()
3.1. Overview of the splitlines() Method
The splitlines()
method splits a string based on newline characters. This is particularly useful when processing file contents line by line or handling data that contains newlines.
text = """apple
banana
cherry"""
lines = text.splitlines()
print(lines) # Output: ['apple', 'banana', 'cherry']
3.2. Retaining Newline Characters
The splitlines()
method provides an option to retain newline characters in the split output. By passing True
as an argument, you can keep the newline characters in the resulting list.
text = """apple\nbanana\ncherry"""
lines = text.splitlines(True)
print(lines) # Output: ['apple\n', 'banana\n', 'cherry']
This approach is useful when you want to preserve the original text format while processing lines individually.
4. Splitting Strings Using Regular Expressions with re.split()
4.1. Basic Usage of re.split()
By using Python’s re
module, you can split strings flexibly with regular expressions. This is particularly useful when you need to split a string using multiple different delimiters.
import re
text = "apple123banana456cherry"
fruits = re.split(r'\d+', text)
print(fruits) # Output: ['apple', 'banana', 'cherry']
4.2. Splitting with Multiple Delimiters
If you need to split a string using multiple delimiters, you can specify them using a regular expression. For example, to use commas, semicolons, and spaces as delimiters, you can do the following:
text = "apple, banana; cherry"
fruits = re.split(r'[;, ]+', text)
print(fruits) # Output: ['apple', 'banana', 'cherry']
4.3. Splitting Based on Complex Patterns
The re.split()
method is highly effective for splitting strings based on specific patterns or rules. For example, you can split a string based on specific numerical patterns or character sequences.
text = "apple100banana200cherry"
fruits = re.split(r'\d+', text)
print(fruits) # Output: ['apple', 'banana', 'cherry']
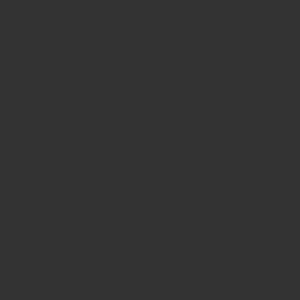
5. Other Useful Methods: partition() and rpartition()
5.1. How to Use the partition() Method
The partition()
method splits a string into three parts based on a specified delimiter. This method is useful when you need to keep the delimiter in the result.
text = "apple@banana@cherry"
parts = text.partition("@")
print(parts) # Output: ('apple', '@', 'banana@cherry')
5.2. Splitting from the Right with rpartition()
The rpartition()
method works similarly to partition()
, but it searches for the delimiter from the right side of the string. This is useful when you want to split based on the last occurrence of a delimiter.
text = "apple@banana@cherry"
parts = text.rpartition("@")
print(parts) # Output: ('apple@banana', '@', 'cherry')
6. Practical Examples: Applying String Splitting in Python
6.1. Handling User Input
When processing user input, you may need to split the entered data into specific formats. The following example splits user input formatted with commas and extracts key-value pairs.
user_input = "name:apple, age:30, city:Tokyo"
info = user_input.split(", ")
for item in info:
key, value = item.split(":")
print(f"{key}: {value}")
6.2. Processing Data from a File
When handling file data, you may need to split it into lines for processing. The following code reads a text file and splits it into a list of lines.
with open('data.txt', 'r') as file:
lines = file.read().splitlines()
print(lines)
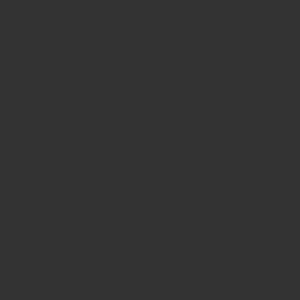
7. Summary: Mastering String Splitting Methods in Python
7.1. Summary
Python provides various methods for splitting strings, including split()
, splitlines()
, re.split()
, and partition()
. Mastering these methods allows for efficient data preprocessing and analysis.
7.2. Next Steps
Try applying these methods in small projects or scripts to find the most suitable approach for different situations.