- 1 1. Introduction
- 2 2. What Is Rounding in Python?
- 3 3. Basic Usage of the round() Function
- 4 4. Precise Rounding with the Decimal Module
- 5 5. Introduction to math.floor() and math.ceil()
- 6 6. Advanced Use Cases: Combining Rounding Methods
- 7 7. Frequently Asked Questions (FAQ)
- 8 8. Summary
- 9 9. Related Articles and References
1. Introduction
Python is a versatile programming language used in various fields, including data analysis and machine learning. One of the most common operations in everyday programming is “rounding numbers.” There are many situations where you need to round a number to a specific decimal place or approximate it to the nearest integer.
This article provides a detailed explanation of various methods for rounding numbers in Python. From the built-in round()
function to the more precise Decimal
module, we will cover different rounding techniques suitable for various scenarios.
By following this guide, you’ll enhance your numerical processing accuracy and improve the reliability of your code.
2. What Is Rounding in Python?
Rounding in Python refers to the process of converting a number to the nearest integer or a specified decimal place. This operation is crucial in data processing and numerical calculations. For example, in financial data or scientific computations where precision is required, how numbers are rounded can significantly impact the results.
Python provides the built-in round()
function for simple rounding tasks. However, this function has some limitations. Floating-point precision can be an issue, and for more accurate results, it is recommended to use Python’s Decimal
module.
In the next section, we’ll explore the basics of rounding with the round()
function.
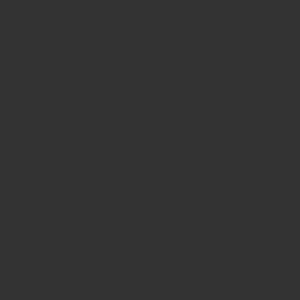
3. Basic Usage of the round()
Function
How to Use round()
The round()
function is a built-in Python function used to round numbers. Here’s a basic example:
print(round(1.5)) # Output: 2
print(round(2.5)) # Output: 2
In this example, both 1.5 and 2.5 are rounded to 2. This happens because Python’s round()
function follows “Bankers’ Rounding,” meaning when a number falls exactly halfway (e.g., 0.5), it is rounded to the nearest even number.
Rounding to a Specific Decimal Place
By providing a second argument to the round()
function, you can specify the number of decimal places to round to:
print(round(1.456, 2)) # Output: 1.46
This example rounds 1.456 to two decimal places.
Limitations of the round()
Function
However, there are some caveats when using round()
. Due to floating-point representation errors, numbers like 0.1 or 0.2 cannot be precisely represented in binary, which may lead to unexpected rounding results. In such cases, using the Decimal
module provides more precise control over numerical accuracy.
In the next section, we’ll take a closer look at the Decimal
module.
4. Precise Rounding with the Decimal Module
What Is the Decimal Module?
Python’s Decimal
module is designed to address floating-point precision issues. It is particularly useful in situations where numerical accuracy is critical, such as financial calculations or scientific computations. The Decimal
module allows for precise decimal arithmetic, avoiding floating-point errors.
For example, using regular floating-point arithmetic can lead to inaccuracies:
print(0.1 + 0.2) # Output: 0.30000000000000004
However, using the Decimal
module ensures accurate calculations:
from decimal import Decimal
print(Decimal('0.1') + Decimal('0.2')) # Output: 0.3
Creating a Decimal
Object
A Decimal
object is created by passing a number as a string, which prevents floating-point representation errors:
from decimal import Decimal
d = Decimal('1.456')
print(d) # Output: 1.456
Rounding with the quantize()
Method
The quantize()
method of a Decimal
object allows rounding to a specified number of decimal places. You can also specify the rounding mode. A common rounding mode is ROUND_HALF_UP
, but other options like ROUND_DOWN
are also available.
Here’s an example that rounds a number to two decimal places:
from decimal import Decimal, ROUND_HALF_UP
d = Decimal('1.456')
rounded = d.quantize(Decimal('0.00'), rounding=ROUND_HALF_UP)
print(rounded) # Output: 1.46
Advantages and Use Cases of the Decimal Module
The Decimal
module is particularly useful in financial applications, where even minor rounding errors can accumulate and lead to significant discrepancies. It is also beneficial in scientific research where precise numerical computations are required.
5. Introduction to math.floor()
and math.ceil()
Using math.floor()
The math.floor()
function returns the largest integer less than or equal to a given number. In other words, it rounds down the number. For example, rounding down 3.9 results in 3.
import math
print(math.floor(3.9)) # Output: 3
The floor()
function is useful when you need to truncate decimal values and work with integer values.
Using math.ceil()
On the other hand, the math.ceil()
function returns the smallest integer greater than or equal to a given number. This is known as “rounding up.” For example, rounding up 3.1 results in 4.
import math
print(math.ceil(3.1)) # Output: 4
When to Use floor()
and ceil()
The floor()
and ceil()
functions are particularly useful in statistical analysis and game development, where specific rounding rules are required. For example, in a point-based system or scoring mechanism, you might need to always round up or down. These functions are also helpful when you need strict rounding behavior that round()
does not provide.
6. Advanced Use Cases: Combining Rounding Methods
Python provides several rounding methods, including round()
, Decimal
, floor()
, and ceil()
. By combining these functions, you can handle numerical processing more flexibly.
Creating a Custom Rounding Function
For example, you can create a custom function that first rounds a number to a specified decimal place and then rounds it up using ceil()
:
import math
def custom_round(value, digits):
rounded_value = round(value, digits)
return math.ceil(rounded_value)
print(custom_round(1.456, 2)) # Output: 2
By implementing a custom function like this, you can achieve specific rounding behaviors that fit your needs. You can also combine it with the Decimal
module for more precise rounding operations.
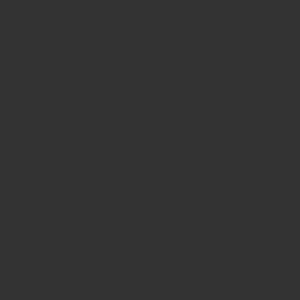
7. Frequently Asked Questions (FAQ)
1. When should I use the round()
function?
The round()
function is the easiest way to round numbers in Python. It is particularly useful for rounding floating-point numbers or integers. However, keep in mind that round()
follows “Bankers’ Rounding” by default, meaning that when a number ends in 0.5, it rounds to the nearest even number.
For example, this code rounds a number to two decimal places using round()
:
print(round(1.456, 2)) # Output: 1.46
2. When should I use the Decimal
module?
The Decimal
module is best suited for situations requiring high numerical accuracy, such as financial calculations and scientific computations. Floating-point arithmetic can introduce small errors, which can accumulate over time. Using Decimal
ensures precise calculations.
For example, this code demonstrates how Decimal
provides an accurate result:
from decimal import Decimal
print(Decimal('0.1') + Decimal('0.2')) # Output: 0.3
3. What should I do if my rounding results are not as expected?
If the rounding results are different from what you expect, consider that the round()
function follows Bankers’ Rounding. When rounding a number ending in 0.5, it rounds to the nearest even number. If you need a different rounding method, use the Decimal
module and specify the rounding mode.
For example, to always round 0.5 up, use ROUND_HALF_UP
:
from decimal import Decimal, ROUND_HALF_UP
d = Decimal('1.25')
print(d.quantize(Decimal('0.1'), rounding=ROUND_HALF_UP)) # Output: 1.3
8. Summary
In this article, we explored various rounding methods in Python. While the round()
function is easy to use, it has limitations in terms of precision and rounding behavior. For more accurate calculations, using the Decimal
module allows finer control over rounding.
Additionally, functions like math.floor()
and math.ceil()
provide alternative rounding approaches that can be useful in specific scenarios. By selecting the appropriate rounding method for your needs, you can improve both the accuracy and reliability of numerical computations in Python.
9. Related Articles and References
To deepen your understanding of rounding in Python, check out the following resources:
- Python Official Documentation –
round()
Function
This is the official Python documentation for theround()
function, explaining its usage and limitations, including Bankers’ Rounding.
Python Official Documentation - Python Official Documentation –
decimal
Module
This documentation covers thedecimal
module, which enables precise numerical calculations and discusses thequantize()
method.
Python Decimal Documentation - Rounding Numbers in Python – note.nkmk.me
A detailed article explaining rounding in Python with code examples, covering bothround()
and thedecimal
module.
How to Round Numbers in Python
By using these resources, you can further enhance your knowledge of numerical rounding and precision handling in Python.