1. What is a Dataclass?
Overview of Dataclass
Python’s dataclass
was introduced in version 3.7 to simplify class definitions and reduce redundant code. It is particularly useful for efficiently defining classes that primarily store data. By using dataclass
, methods such as __init__
and __repr__
, which are commonly written in classes, can be automatically generated.
For example, in a traditional class definition, you would need to manually define an initializer method, but with dataclass
, it becomes much more concise:
from dataclasses import dataclass
@dataclass
class User:
name: str
age: int
With the above code, the __init__
and __repr__
methods are automatically generated, making it easy to define a class focused on data storage. Additionally, by using type annotations, you can clearly specify the data types and structure of the class, improving code readability.
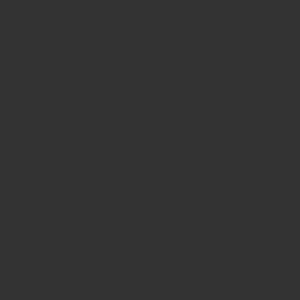
2. Benefits of Dataclass
Simplified Code
Using dataclass
significantly reduces the amount of code compared to traditional class definitions, making it easier to read. The automatic generation of methods like __init__
and __repr__
eliminates the need to write them manually, reducing potential errors.
@dataclass
class Product:
id: int
name: str
price: float
Even for a simple class like this, dataclass
automatically provides functionality such as initialization and string representation. Additionally, if you need to add more fields later, modifications are straightforward, offering great flexibility.
Automatically Generated Methods
Besides the __init__
method, dataclass
also automatically generates methods like __repr__
and __eq__
. This allows for easy object comparisons between classes and simplifies converting object states into string representations without writing additional code.
Default Values and Type Annotations
dataclass
allows you to set default values for fields and supports type annotations. This enables developers to clearly specify data types and initial values, making class definitions more intuitive.
@dataclass
class Employee:
name: str
age: int = 25 # Default age is 25
By setting default values for fields, you can define parameters that can be omitted during initialization as needed.
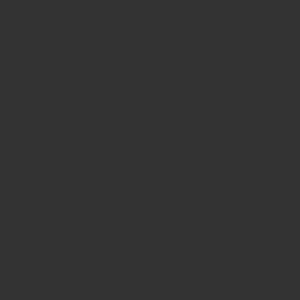
3. Comparison with Traditional Class Definitions
Memory and Performance Optimization
Compared to traditional class definitions, dataclass
also has advantages in terms of memory usage and performance. Especially in applications handling large amounts of data, using the slots
option, introduced in Python 3.10, can further optimize memory efficiency.
@dataclass(slots=True)
class User:
name: str
age: int
By specifying slots=True
, instances use memory-efficient slots instead of generating dictionary objects, reducing memory consumption when handling many instances. Additionally, attribute access becomes faster, improving performance.
Differences from Traditional Classes
In traditional class definitions, all methods must be defined manually. However, with dataclass
, these methods are generated automatically, allowing developers to focus on designing data structures. When dealing with classes that have many fields or require specific behaviors, dataclass
helps keep the code concise and maintainable.
4. Advanced Features of Dataclass
Memory Optimization with slots
Starting with Python 3.10, dataclass
supports slots
, which further optimizes memory usage. Using __slots__
stores instance attributes in a lightweight structure instead of a dictionary, reducing memory consumption.
Let’s take a look at an example to see its effect:
@dataclass(slots=True)
class Person:
name: str
age: int
When using this class with large datasets, memory consumption is significantly reduced. Additionally, since dynamic attribute addition is disabled with slots, unintended bugs can be prevented.
Creating Immutable Classes (frozen=True
)
The dataclass
option frozen=True
allows you to define immutable classes whose attributes cannot be changed after creation. Immutable objects are useful in scenarios requiring data consistency or in thread-safe applications.
@dataclass(frozen=True)
class ImmutableUser:
username: str
age: int
With frozen=True
, attempting to modify an attribute after creation will raise an AttributeError
, ensuring data immutability.
Custom Fields and the field()
Function
Additionally, dataclass
allows fine control over fields using the field()
function. This is useful when you want to ignore specific fields during initialization or set complex default values.
@dataclass
class Product:
name: str
price: float = field(default=0.0, init=False)
In this example, the price
field is not included during initialization and defaults to 0.0. This provides flexibility in handling class behavior under specific conditions.
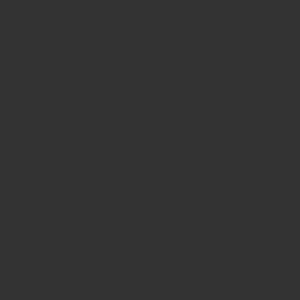
5. Practical Use Cases of Dataclass
Managing User Data
dataclass
is highly suitable for classes primarily used for data storage. For example, it can be used to define classes for storing user data or configuration settings concisely.
@dataclass
class UserProfile:
username: str
email: str
is_active: bool = True
Even when handling classes with many fields, using dataclass
keeps the code readable and easy to maintain.
Data Transformation and JSON Handling
dataclass
is also convenient for data transformation and JSON handling. It allows easy mapping of data retrieved from a database or API into class objects and seamless conversion to other formats. Additionally, Python’s built-in dataclasses
module provides functions to convert objects into tuples or dictionaries.
import json
from dataclasses import dataclass, asdict
@dataclass
class Product:
id: int
name: str
price: float
product = Product(1, "Laptop", 999.99)
print(json.dumps(asdict(product)))
In this example, the asdict()
function converts a dataclass
object into a dictionary, which is then output as JSON. This feature makes it easy to handle data in various formats while keeping it structured as class objects.
6. Integration with Other Libraries
Data Validation with Pydantic
dataclass
can be integrated with other Python libraries, especially Pydantic
, to enhance data validation. Pydantic
is a library that uses type hints to easily add validation logic to classes, ensuring data accuracy.
The following example demonstrates how to add type validation to a dataclass
using Pydantic
:
from pydantic.dataclasses import dataclass
from pydantic import ValidationError
@dataclass
class Book:
title: str
pages: int
try:
book = Book(title=123, pages="two hundred")
except ValidationError as e:
print(e)
In this code, an error occurs if the title
field is not a string or if pages
is not an integer. By incorporating validation into dataclass
, you can ensure accurate data handling, making it ideal for large-scale applications and API development.
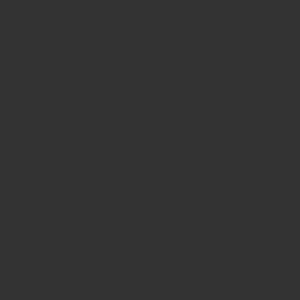
7. Common Mistakes When Using Dataclass
Mutable Default Arguments
One common mistake when using dataclass
is setting a mutable object as a default argument. For example, if a list or dictionary is used as a default value, all instances may share the same object.
from dataclasses import dataclass, field
@dataclass
class Team:
members: list = field(default_factory=list)
By using default_factory
, you can ensure that each instance gets its own list, avoiding unintended behavior. Avoiding mutable default arguments is crucial to prevent unexpected bugs.
Mismatch Between Attribute Types and Default Values
Another common mistake is setting a default value that does not match the declared type. While type annotations are recommended in dataclass
, mismatches between types and default values can lead to errors.
@dataclass
class User:
name: str
age: int = "twenty" # Incorrect
To prevent such issues, ensure that the default values match the specified type annotations.
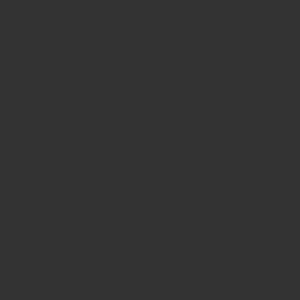
8. Conclusion
Python’s dataclass
simplifies the definition of classes focused on data storage while offering numerous benefits to developers. In addition to improving code readability, it supports memory optimization with slots
and guarantees data immutability with the frozen
option, making it suitable for a wide range of use cases. Furthermore, its compatibility with other libraries enables advanced functionalities like data validation and JSON conversion, making it an excellent choice for large-scale application development.
Considering these advantages, try incorporating dataclass
in your next project!