- 1 1. What is argparse? Basic Usage in Python
- 2 2. Why Use argparse? The Best Choice for CLI Tools
- 3 3. Basic Structure of argparse: Step-by-Step Guide
- 4 4. Example: A Simple Python Program Using argparse
- 5 5. Key Features of argparse You Should Know
- 6 6. Error Handling and Debugging in argparse
- 7 7. Real-World Applications of argparse
- 8 8. Conclusion
1. What is argparse? Basic Usage in Python
What is argparse?
argparse
is a standard Python library designed for handling command-line arguments. It allows users to easily specify command-line arguments when executing a program, parse them, and customize the program’s behavior flexibly. For example, users can specify file paths or configuration options via the command line, making it easier to create user-friendly CLI tools.
import argparse
parser = argparse.ArgumentParser(description="File processing program")
parser.add_argument("input_file", help="Path to the file to be processed")
args = parser.parse_args()
print(f"Processing file: {args.input_file}")
In this code, the user provides a file path as input_file
via the command line, which is then displayed. The process is simple: define arguments with add_argument
and parse them using parse_args()
.
2. Why Use argparse? The Best Choice for CLI Tools
What is a CLI Tool?
A CLI (Command Line Interface) tool is a program that is operated directly from the command line, making it particularly useful for system administration, data processing, and automation. When creating a CLI tool in Python, argparse
is extremely useful because it automates argument handling, error handling, and help message generation.
Benefits of argparse
- Flexible Argument Configuration: Easily define required and optional arguments, allowing you to design a user-friendly program.
- Automatic Error Handling: If arguments are missing or invalid values are provided,
argparse
automatically generates an error message. - Help Messages: By default,
argparse
provides a--help
option, making it easy for users to check how to use the program.
The following example demonstrates how the --help
option displays usage instructions for a CLI tool:
$ python script.py --help
usage: script.py [-h] input_file
positional arguments:
input_file File to be processed
optional arguments:
-h, --help show this help message and exit
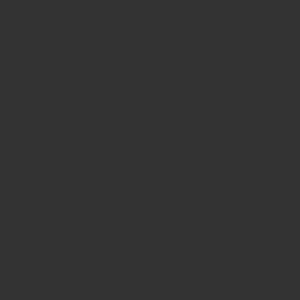
3. Basic Structure of argparse: Step-by-Step Guide
Creating an ArgumentParser
To parse arguments, first, create an ArgumentParser
object. This object defines the program’s description and usage.
parser = argparse.ArgumentParser(description="File processing program")
Adding Arguments
Next, use add_argument()
to add arguments. You can specify whether an argument is required or optional. Additionally, you can add a description using the help
parameter.
parser.add_argument("input_file", type=str, help="Specify the input file")
parser.add_argument("--verbose", action="store_true", help="Enable detailed output")
Parsing Arguments
Finally, use the parse_args()
method to parse the arguments. The provided command-line arguments will be stored in the args
object.
args = parser.parse_args()
4. Example: A Simple Python Program Using argparse
Basic Example
The following is a simple example using argparse
to create a CLI tool that accepts a filename and an optional verbose mode.
import argparse
parser = argparse.ArgumentParser(description="File processing program")
parser.add_argument("file", help="Specify the file path")
parser.add_argument("--verbose", action="store_true", help="Enable detailed output")
args = parser.parse_args()
if args.verbose:
print(f"Processing file '{args.file}' in verbose mode")
else:
print(f"Processing file '{args.file}'")
This program uses the --verbose
option to switch between detailed output mode and normal mode.
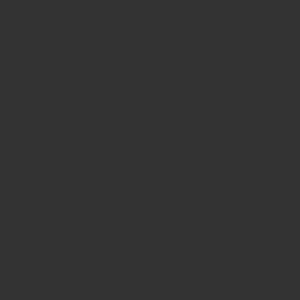
5. Key Features of argparse You Should Know
Mutually Exclusive Arguments
Mutually exclusive arguments ensure that certain arguments cannot be used together. For example, if you want to prevent both --foo
and --bar
from being specified simultaneously, you can use this feature.
parser = argparse.ArgumentParser(description="Example of mutually exclusive arguments")
group = parser.add_mutually_exclusive_group()
group.add_argument("--foo", action="store_true", help="Enable foo")
group.add_argument("--bar", action="store_true", help="Enable bar")
args = parser.parse_args()
Subcommands
Using subcommands allows you to organize a program’s functionality into multiple actions. For example, you can use install
and uninstall
as different commands within the same program.
parser = argparse.ArgumentParser(description="Example of subcommands")
subparsers = parser.add_subparsers(dest="command")
install_parser = subparsers.add_parser('install')
install_parser.add_argument('package', help="Name of the package to install")
uninstall_parser = subparsers.add_parser('uninstall')
uninstall_parser.add_argument('package', help="Name of the package to uninstall")
args = parser.parse_args()
if args.command == "install":
print(f"Installing package {args.package}")
elif args.command == "uninstall":
print(f"Uninstalling package {args.package}")
6. Error Handling and Debugging in argparse
Automatic Error Messages
argparse
automatically generates error messages when required arguments are missing or invalid values are provided.
$ python script.py
usage: script.py [-h] --input INPUT
script.py: error: the following arguments are required: --input
Custom Error Messages
Sometimes, the default error messages may not be sufficient. By adding custom messages, you can provide clearer feedback.
parser = argparse.ArgumentParser(description="Example of custom error messages")
parser.add_argument("--input", required=True, help="Specify the input file")
try:
args = parser.parse_args()
except argparse.ArgumentError as err:
print(f"Error: {err}")
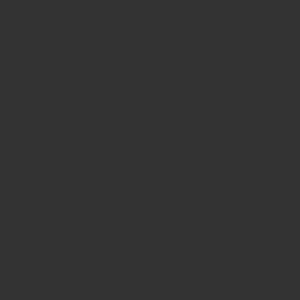
7. Real-World Applications of argparse
Using argparse in Automation Tools
argparse
is ideal for automation tools involving file processing and data analysis. Users can specify file paths and processing modes via the command line, allowing for flexible tool design.
$ python data_processor.py --input data.csv --output results.json --verbose
Using argparse in Large-Scale Projects
For large-scale projects, combining subcommands and mutually exclusive arguments improves usability. For instance, package management systems often use commands like install
and remove
within a single tool.
$ python package_manager.py install package_name
By leveraging argparse
, you can create user-friendly systems while improving code reusability and maintainability.
8. Conclusion
By using argparse
, you can implement a flexible and powerful command-line interface (CLI) in your Python programs. Features like required and optional arguments, subcommands, and mutually exclusive arguments make it easier to design user-friendly and manageable programs. This is especially useful in large-scale projects and automation scenarios.
Understanding real-world applications and performance considerations allows you to optimize your programs further. Additionally, implementing error handling and best practices enables you to create tools that help users identify errors more efficiently.
If you have any questions, feel free to ask!