1. What is a Global Variable?
Basic Concept of Global Variables
In Python, a global variable is a variable that can be accessed throughout the entire program. It is defined outside of functions and classes and is used to share data between different functions and modules. However, excessive use of global variables can lead to unexpected bugs, so caution is required when using them.
# Example of a global variable
global_var = "Global Variable"
def show_global():
print(global_var)
show_global() # Output: Global Variable
Global variables are defined outside functions and classes and can be referenced from anywhere in the program. However, special care is needed when modifying them.
Difference Between Local and Global Variables
Unlike global variables, local variables are defined within a function, and their scope is limited to that function. Local variables are discarded when the function ends and cannot be accessed from other functions or externally.
def example_func():
local_var = "Local Variable"
print(local_var)
example_func() # Output: Local Variable
# print(local_var) # Error: Local variables cannot be accessed from outside the function
Understanding the difference between local and global variables and using them appropriately improves code maintainability.
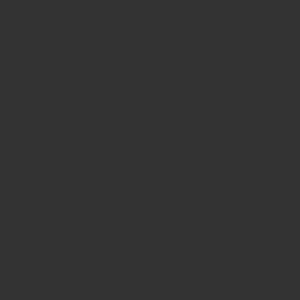
2. Using Global Variables Inside Functions
Referencing and Modifying Global Variables
Global variables can be referenced inside functions, but modifying their value within a function requires the global
keyword. Without this keyword, a local variable with the same name will be created inside the function, leaving the global variable unchanged.
counter = 0 # Global variable
def increase_counter():
global counter
counter += 1
increase_counter()
print(counter) # Output: 1
Introduction to the nonlocal
Keyword
The nonlocal
keyword is used to modify an enclosing function’s local variable in nested functions. This is useful when using closures or maintaining a state within a function.
def outer_func():
outer_var = "Outer"
def inner_func():
nonlocal outer_var
outer_var = "Modified Outer"
inner_func()
print(outer_var)
outer_func() # Output: Modified Outer
Using nonlocal
allows inner functions to access and modify the outer function’s local variables.
3. Precautions When Using Global Variables
Risks of Overusing Global Variables
While global variables are convenient, excessive use can lead to unintended consequences across the program. If multiple functions modify the same global variable, it becomes difficult to track which function changed the value and when.
counter = 0
def increment():
global counter
counter += 1
def decrement():
global counter
counter -= 1
increment()
decrement()
print(counter) # Output: 0
Since global variables can be modified in multiple places, bugs may arise. Therefore, it is recommended to minimize their use and manage data through alternative methods.
Error Handling and Debugging
Unexpected errors can occur when using global variables. Adding error handling and utilizing the logging
module can help identify where errors occur, making debugging easier.
import logging
logging.basicConfig(level=logging.DEBUG)
counter = 0
def increment():
global counter
try:
counter += 1
logging.debug(f"Counter incremented: {counter}")
except Exception as e:
logging.error(f"Error: {e}")
increment()
This approach helps track changes to global variables and log any errors that may occur.
4. Best Practices for Using Global Variables
Minimizing the Use of Global Variables
It is recommended to minimize the use of global variables whenever possible. Instead, passing data through function arguments and return values helps maintain clear data flow and prevents unintended modifications.
def add_points(score, points):
return score + points
current_score = 0
current_score = add_points(current_score, 10)
print(current_score) # Output: 10
By using function arguments and return values, data can be managed without relying on global variables.
Managing State Using Classes
Using classes can help manage state without relying on global variables. Classes provide a structured way to encapsulate data and maintain clear scopes.
class Game:
def __init__(self):
self.score = 0
def add_points(self, points):
self.score += points
game = Game()
game.add_points(10)
print(game.score) # Output: 10
By using classes, state is confined to specific instances, preventing unintended modifications from other parts of the program.
5. Practical Examples and Applications
Example Use in Game Development
In game development, global variables are sometimes used to manage the state of the score or player lives. However, for large-scale projects, managing states using classes or databases is recommended.
score = 0 # Global variable
def increase_score(points):
global score
score += points
increase_score(10)
print(f"Player Score: {score}") # Output: Player Score: 10
Using Global Variables for Configuration Management
Global variables are often used to store application-wide settings, such as debug mode or version information. However, integrating them with configuration files or environment variables allows for more efficient management.
config = {
'debug': True,
'version': '1.0'
}
def print_config():
print(f"Debug Mode: {config['debug']}, Version: {config['version']}")
print_config() # Output: Debug Mode: True, Version: 1.0
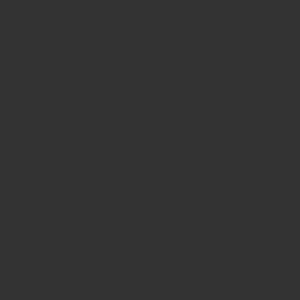
6. Summary and Next Steps
Global variables can be useful in simple scenarios, but excessive use can make code more complex and lead to bugs. Utilizing function arguments, return values, and classes instead of global variables contributes to efficient and safer program design.
Topics to Learn Next
As the next step, it is recommended to learn about closures, module scope, and object-oriented programming (OOP). These concepts will help design more complex programs without relying on global variables.
External Resources and References
- Python Official Documentation
Provides detailed explanations about scope, closures, and OOP in Python. Understanding the LEGB rule (Local, Enclosing, Global, Built-in) is particularly useful.
Python Official Documentation