1. What is the type()
Function in Python?
Overview of the type()
Function
In Python, the type()
function is frequently used to check the data type of an object. This function returns the type of the given object as an argument, making it a useful tool for debugging and type checking. By leveraging Python’s dynamic typing, you can easily determine what type of data an object holds.
Basic Usage
The type()
function is used as follows:
type(object)
This function returns the object’s type as a class. For example, you can check the type of an integer or a string as shown below:
print(type(123)) # Output: <class 'int'>
print(type("Python")) # Output: <class 'str'>
By using the type()
function, you can clearly identify an object’s type, which is important before performing type-dependent operations.
2. Basic Usage of the type()
Function
Checking Basic Data Types
Python has several basic data types that can be checked using type()
. Here are some common examples:
print(type(3.14)) # Output: <class 'float'>
print(type(True)) # Output: <class 'bool'>
These fundamental data types are frequently used in Python. By using the type()
function, you can verify variable types during program execution to prevent type-related errors.
Using type()
in Conditional Statements
The type()
function can be used in conditional statements to check whether an object belongs to a specific type. For example, to check if an object is an integer, you can use:
if type(123) is int:
print("This is an integer.")
Additionally, you can check for multiple types using the in
operator:
if type([1, 2, 3]) in (list, int):
print("This is either a list or an integer.")
Using type checks in conditional statements helps prevent unexpected errors in your program.
3. Checking Complex Data Types
Checking the Type of Lists, Tuples, and Dictionaries
In Python, you can also check the type of complex data structures such as lists, tuples, and dictionaries using type()
. Below are examples for each data type:
my_list = [1, 2, 3]
print(type(my_list)) # Output: <class 'list'>
my_tuple = (1, 2, 3)
print(type(my_tuple)) # Output: <class 'tuple'>
my_dict = {'a': 1, 'b': 2}
print(type(my_dict)) # Output: <class 'dict'>
These complex data types are essential for storing and managing multiple data elements efficiently in Python programs.
Checking the Type of Sets and Frozen Sets
The type()
function can also determine whether an object is a set (set
) or a frozen set (frozenset
). Here are examples:
my_set = {1, 2, 3}
print(type(my_set)) # Output: <class 'set'>
my_frozenset = frozenset([1, 2, 3])
print(type(my_frozenset)) # Output: <class 'frozenset'>
These data types are useful when performing set operations under specific conditions.
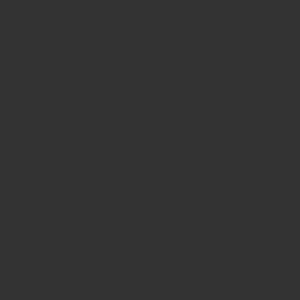
4. Type Conversion and Applications of type()
in Python
Overview of Type Conversion
Python provides several built-in functions for converting data types. Functions such as int()
, float()
, and str()
allow you to convert values between different types, such as from a string to an integer or a floating-point number.
my_str = "100"
my_int = int(my_str)
print(type(my_int)) # Output: <class 'int'>
Using type conversion effectively, along with type()
, helps ensure that data is in the correct format for further processing.
Verifying Type After Conversion
The type()
function is useful for verifying whether a type conversion was performed correctly. For instance, you can check the result of converting a string to a floating-point number:
my_float = float("3.14")
print(type(my_float)) # Output: <class 'float'>
Type conversion is especially useful when handling user input or reading data from files, ensuring that the data is in the expected format.
5. Differences Between type()
and isinstance()
Features of isinstance()
Python provides the isinstance()
function, which is similar to type()
. This function checks whether an object is an instance of a specified class or its subclass.
x = 5
if isinstance(x, int):
print("x is an integer.")
Unlike type()
, isinstance()
takes class inheritance into account, making it useful when checking for subclass relationships.
When to Use type()
vs. isinstance()
The type()
function performs a strict type check and ignores subclass relationships. On the other hand, isinstance()
recognizes both the specified type and its subclasses. When dealing with objects that have an inheritance structure, using isinstance()
is recommended.
class Animal:
pass
class Dog(Animal):
pass
dog = Dog()
print(isinstance(dog, Animal)) # True
print(type(dog) is Animal) # False
In this example, since the Dog
class inherits from the Animal
class, isinstance()
recognizes dog
as an instance of Animal
. However, type()
strictly identifies dog
as an instance of the Dog
class.
6. Summary and Key Points
The type()
function in Python is a highly useful tool for checking object types. However, when dealing with objects that have an inheritance structure, isinstance()
may be more appropriate. Additionally, when using type()
, it is essential to implement proper error handling, especially in dynamic typing scenarios where data types may change.
Type checking and conversion play a crucial role in data processing and user input validation. By effectively utilizing these functions, you can create more robust and error-resistant programs.