1. What is Python’s time Module?
1.1 Overview of the time Module
Python’s time
module is a standard library that provides functions for handling time and date-related operations within a program.
This module retrieves the number of seconds elapsed since the epoch (January 1, 1970, 00:00:00 UTC) based on the system clock. It is commonly used for adding timestamps to log files and measuring program performance.
One of the key advantages of the time
module is its simplicity. It requires no complex setup, making it ideal for basic time management and measurement tasks.
1.2 Key Features
The time
module offers various time-related functions. Some of the most commonly used ones include:
time.time()
: Returns the number of seconds elapsed since the epoch as a floating-point value.time.sleep(secs)
: Pauses program execution for the specified number of seconds. Useful for temporarily halting a process.time.ctime()
: Converts a timestamp into a human-readable format.
By utilizing these functions, you can perform a wide range of time-related operations, from simple time retrieval to advanced performance measurements.
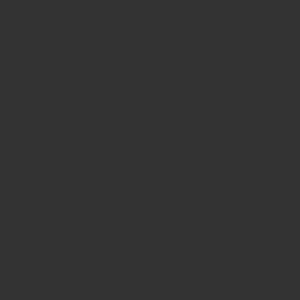
2. How to Retrieve the Current Time
2.1 Using time.time()
to Get the Current Time
The time.time()
function returns the number of seconds that have passed since the epoch. This is the simplest method for retrieving the current time. See the example below:
import time
current_time = time.time()
print(current_time)
This code prints the current epoch time (a floating-point number representing the number of seconds). While epoch time is useful for internal calculations, it is not intuitive for humans. In the next section, we’ll explore how to format it using strftime()
.
2.2 Converting Time to a Human-Readable Format with strftime()
The time.strftime()
function is used to format epoch time into a more readable date and time representation. This is especially useful for displaying timestamps in log files or on user interfaces.
The following example prints the current time in the format “Year-Month-Day Hour:Minute:Second”:
import time
formatted_time = time.strftime('%Y-%m-%d %H:%M:%S', time.localtime())
print(formatted_time)
In this example, time.localtime()
converts the current timestamp to local time, and strftime()
formats it.
– %Y
: Four-digit year
– %m
: Month
– %d
: Day
By using this method, you can easily present time data in a user-friendly format.
3. Measuring Execution Time
3.1 Basic Execution Time Measurement with time.time()
One of the simplest ways to measure execution time is by using time.time()
. This method captures the start and end times of a process and calculates the duration.
import time
start_time = time.time()
# Example: Loop process
for i in range(1000000):
pass
end_time = time.time()
print(f"Execution time: {end_time - start_time} seconds")
In this example, we measure the time taken for a loop to execute. Since time.time()
measures time in seconds, it may not be highly precise but is sufficient for simple performance measurements.
3.2 High-Precision Measurement with perf_counter()
For more precise measurements, use perf_counter()
. This function utilizes the system’s performance counter, offering higher precision.
import time
start_time = time.perf_counter()
# High-precision measurement
for i in range(1000000):
pass
end_time = time.perf_counter()
print(f"High-precision execution time: {end_time - start_time} seconds")
perf_counter()
provides nanosecond-level accuracy, making it ideal for time-critical applications and performance optimizations.
3.3 Benchmarking with the timeit
Module
If you need to benchmark performance, the timeit
module is highly useful. It repeatedly runs a specific operation and calculates the average execution time.
import timeit
print(timeit.timeit('"-".join(str(n) for n in range(100))', number=10000))
This code measures the execution time of joining list elements as a string over 10,000 iterations.
timeit
minimizes external noise, allowing for more accurate performance comparisons.
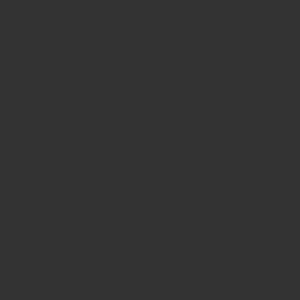
4. Date Handling: Comparison with the datetime Module
4.1 Retrieving the Current Time with datetime.now()
Alongside the time
module, Python’s datetime
module is highly useful, particularly for working with date calculations.
from datetime import datetime
now = datetime.now()
print(now)
This code retrieves the current date and time as a datetime
object.
Since the datetime
module simplifies time calculations and timezone management, it is preferred for more complex date-related tasks.
4.2 Choosing Between the time
and datetime
Modules
While the time
module is simple and great for high-precision time measurement, the datetime
module is better suited for handling date-related operations.
For example, if your application involves working with specific dates or performing date-based calculations, the datetime
module is the more appropriate choice.
5. Practical Examples: Using the time Module
5.1 Adding Timestamps to Logs
Timestamps are commonly added to log files to track events within a program. The time.ctime()
function makes it easy to obtain the current timestamp and record it in a log.
import time
log_entry = f"{time.ctime()} - Error message"
print(log_entry)
This example uses time.ctime()
to generate a human-readable timestamp for logging events.
5.2 Optimizing Program Performance
Time measurement is useful for performance optimization. By measuring execution time with the time
module or timeit
module, you can identify bottlenecks and optimize code execution speed.
For example, if two different functions achieve the same result, measuring their execution times can help you choose the more efficient approach.
6. Conclusion
Python’s time
module provides a simple and effective way to manage time-related operations. From basic tasks like measuring execution time and adding timestamps to logs to advanced techniques using perf_counter()
and the timeit
module, it offers a wide range of functionalities.
For performance optimization and time-sensitive tasks, the time
module is a powerful and lightweight tool. Additionally, when working with complex date operations, combining it with the datetime
module allows for better time zone handling and detailed date calculations. Understanding the strengths of each module and using them appropriately will enhance the efficiency and practicality of your Python programs.