- 1 1. What is Python’s type() Function?
- 2 2. Basic Usage Examples of the type() Function
- 3 3. The Difference Between type() and isinstance()
- 4 4. Practical Applications of the type() Function
- 5 5. Common Data Types in Python and Checking Them with type()
- 6 6. Advanced Uses of the type() Function
- 7 7. Best Practices for Using type()
- 8 8. Summary
1. What is Python’s type() Function?
The Basics of type() in Python
The type()
function in Python is a useful tool for determining the data type of an object. Checking data types is essential for understanding how a program operates. In dynamically typed languages like Python, the type of a variable directly affects how the code behaves, making type()
an important function.
Basic Usage
For example, if you want to check the data type of a string or a number, you can use the following code:
print(type("Hello")) # <class 'str'>
print(type(123)) # <class 'int'>
This code returns the data type of each object. The type()
function is very useful when you need to confirm that data types are as expected.
Why Checking Data Types is Important
There are many situations where checking data types is necessary. For instance, verifying user input ensures that the correct data type is used, preventing errors and unexpected behavior. Additionally, understanding data types makes debugging and maintaining code much easier.
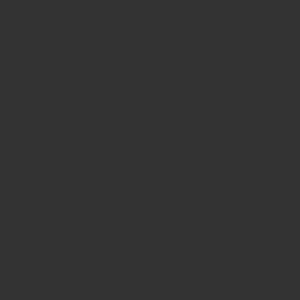
2. Basic Usage Examples of the type() Function
Using type() to Check Data Types
Let’s look at some specific examples. The type()
function can also be used to check complex data types like lists and dictionaries.
print(type([1, 2, 3])) # <class 'list'>
print(type({"key": "value"})) # <class 'dict'>
By using type()
, you can easily determine whether an object is a list, dictionary, or another data structure. This allows you to write logic that handles different data types appropriately.
Using Type Checking in Conditional Statements
You can also use type checking to control the flow of a program. The following example checks whether a variable is an integer before proceeding:
num = 100
if type(num) is int:
print("This is an integer")
By incorporating type checking into conditions, you can ensure that appropriate actions are taken based on the data type.
3. The Difference Between type() and isinstance()
Choosing Between isinstance() and type()
The type()
function returns the exact type of an object, but Python also provides another useful function: isinstance()
. This function checks whether an object is an instance of a specific class or one of its subclasses.
How to Use isinstance()
For example, if a Dog
class inherits from an Animal
class, isinstance()
will take this inheritance into account.
class Animal: pass
class Dog(Animal): pass
dog = Dog()
print(isinstance(dog, Animal)) # True
In this case, dog
is an instance of the Dog
class, but it is also recognized as an instance of the Animal
class. While type()
ignores inheritance, isinstance()
considers it, making it useful for flexible type checking.
When to Use Each Function
Generally, if you need strict type checking, use type()
. If you need to check for a specific class or any of its subclasses, isinstance()
is the better choice. In object-oriented programming, isinstance()
is often more practical.
4. Practical Applications of the type() Function
Using type() for Conditional Processing Based on Data Types
The type()
function is useful when you need to execute different actions based on data types. For example, you can filter elements in a list based on their type.
mylist = [1, "two", 3.0, [4, 5], {"six": 6}]
only_numbers = [x for x in mylist if type(x) in (int, float)]
print(only_numbers) # [1, 3.0]
In this example, only integers and floats are extracted from the list. This allows efficient handling of mixed data types.
Using type() for Debugging and Data Validation
Checking data types is a crucial part of development, especially when handling external data input. If unexpected types appear in your program, type()
can help identify the issue and prevent errors, improving code reliability.
5. Common Data Types in Python and Checking Them with type()
Common Data Types in Python
Python has various built-in data types. Here are some of the most commonly used ones:
print(type("Hello")) # <class 'str'>
print(type(123)) # <class 'int'>
print(type(12.3)) # <class 'float'>
print(type([1, 2, 3])) # <class 'list'>
print(type({"key": "value"})) # <class 'dict'>
print(type((1, 2))) # <class 'tuple'>
Checking the Type of Custom Classes
Python allows you to use type()
to check the type of user-defined classes as well.
class MyClass:
pass
obj = MyClass()
print(type(obj)) # <class '__main__.MyClass'>
This is useful for debugging and understanding your code, especially when working with custom classes.
6. Advanced Uses of the type() Function
Dynamically Creating Classes with type()
The type()
function can do more than just return an object’s type—it can also be used to dynamically create new classes. This is useful for more advanced programming techniques, such as metaprogramming.
MyClass = type('MyClass', (object,), {'x': 5})
obj = MyClass()
print(obj.x) # 5
In this example, a new class called MyClass
is created dynamically at runtime. This technique is particularly useful in large-scale applications and frameworks where flexible class creation is required.
7. Best Practices for Using type()
Using isinstance() for More Flexible Type Checking
While type()
is a powerful function, using isinstance()
in certain situations provides more flexibility. This is especially true when working with class inheritance, where isinstance()
can recognize instances of subclasses.
Avoiding Over-Reliance on Data Types
Relying too much on strict type checking can limit the flexibility of your code. In cases where new types or classes might be introduced in the future, it’s best to design your code to handle various types dynamically. This helps improve code maintainability and scalability.
8. Summary
Mastering the type() Function
The type()
function is an essential tool in Python for checking data types. It is particularly useful for debugging, validating data types, and improving code maintainability. Understanding its basic usage and advanced applications can make your programs more flexible and reliable.
Key Takeaways
Through this article, you have learned:
– How to use the type()
function to check data types.
– The difference between type()
and isinstance()
.
– Practical use cases for type checking.
By incorporating type checking into your code, you can prevent errors and write more reliable programs.
Next Steps
Now that you understand how type()
and isinstance()
work, try applying them in your own projects. Even in small projects, verifying data types can help prevent errors. If you’re working with complex classes or data structures, these functions will improve the readability and maintainability of your code.