- 1 1. Understanding the Basics of the with Statement
- 2 2. Basic Usage of the with Statement
- 3 3. File Operation Modes in the with Statement
- 4 4. Handling Multiple Files Simultaneously
- 5 5. Benefits of Using the with Statement
- 6 6. Practical Examples and Best Practices
- 7 7. Advanced Usage of the with Statement in Python 3.3+
- 8 8. Enhancements to the with Statement in Python 3.9+
- 9 9. Precautions When Using the with Statement
- 10 10. Conclusion
1. Understanding the Basics of the with
Statement
The Python with
statement is a syntax feature designed to simplify resource management. It is commonly used for operations that involve opening and closing resources, such as file handling, network connections, and database connections. By using the with
statement, resources are automatically released (closed) after use, making the code cleaner and reducing the risk of errors.
What is the with
Statement?
The Python with
statement utilizes a mechanism called “context managers” to handle the opening and closing of resources automatically. Typically, when opening a file, the open()
function is used, and the file must be closed afterward using the close()
method. However, by using the with
statement, this entire process can be written in a single line, ensuring the file is closed automatically and making the code more concise.
with open('example.txt', 'r') as file:
content = file.read()
In the above example, the file is opened, read, and then automatically closed. The with
statement simplifies resource management and enhances code readability.
2. Basic Usage of the with
Statement
Using the with
statement eliminates the need to explicitly open and close resources, resulting in cleaner code. Let’s look at a basic example of file handling, where a file is opened, read, and its contents are displayed.
Example: File Handling with the with
Statement
The following code demonstrates a simple way to read a file using the with
statement.
with open('sample.txt', 'r') as file:
content = file.read()
print(content)
In this code, the open()
function opens the file, and the as
keyword assigns the file object to file
. The read()
method reads the file’s contents, which are then printed using the print()
function. By using the with
statement, there is no need to explicitly call close()
, as the resource is automatically released.
Comparison: Without the with
Statement
Without the with
statement, the file must be manually closed.
file = open('sample.txt', 'r')
content = file.read()
print(content)
file.close()
In this approach, after opening the file with open()
, the close()
method must be explicitly called to close it after processing. Using the with
statement automates this closing process, making the code safer and more efficient.
3. File Operation Modes in the with
Statement
When opening a file with the with
statement, you need to specify the file mode. The most commonly used modes are read mode ('r'
), write mode ('w'
), and append mode ('a'
).
Explanation of Each Mode
'r'
: Read mode. Opens the file for reading. If the file does not exist, an error occurs.'w'
: Write mode. Opens the file for writing. If the file does not exist, a new file is created. If the file already exists, it is overwritten.'a'
: Append mode. Opens the file to add content at the end. If the file does not exist, a new file is created.
Examples of Writing and Appending
Here are examples of writing data to a file using the with
statement.
# Create a new file and write to it
with open('sample.txt', 'w') as file:
file.write('Hello, world!\n')
# Open the file in append mode
with open('sample.txt', 'a') as file:
file.write('This is an additional line.\n')
In this example, the file is first opened in 'w'
mode to create and write content. Then, it is reopened in 'a'
mode to append additional content.
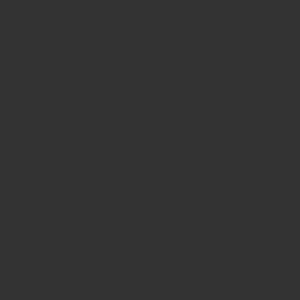
4. Handling Multiple Files Simultaneously
The with
statement allows multiple files to be handled simultaneously. There are two methods to achieve this: nesting with
statements and using a comma-separated approach.
Using Nested with
Statements
This method involves nesting multiple with
statements.
with open('file1.txt', 'r') as file1:
with open('file2.txt', 'r') as file2:
content1 = file1.read()
content2 = file2.read()
print(content1, content2)
While intuitive, deep nesting can make the code harder to read.
Using a Comma-Separated with
Statement
This approach combines multiple file operations in a single line using commas.
with open('file1.txt', 'r') as file1, open('file2.txt', 'r') as file2:
content1 = file1.read()
content2 = file2.read()
print(content1, content2)
This method makes the code more concise, but if too many files are opened, the line may become too long. In such cases, adding line breaks can improve readability.
5. Benefits of Using the with
Statement
The with
statement not only improves code readability but also provides significant functional advantages.
Preventing Errors with Automatic Resource Closing
The biggest advantage of the with
statement is its ability to automatically release resources. In file operations, forgetting to call the close()
method can lead to errors. This is especially important in large-scale projects or long scripts where ensuring proper resource management improves reliability.
Improved Code Readability
The with
statement groups resource operations into a single block, making it clear where the process begins and ends. This makes it easier for other developers to understand that resources are opened and closed automatically within the with
block.
Reducing Human Errors
Using the with
statement helps prevent mistakes such as forgetting to close resources or mismanaging them. This is particularly useful in complex operations where resource management is crucial, ensuring safe and efficient code execution.
6. Practical Examples and Best Practices
Finally, let’s explore some practical examples and best practices for using the with
statement.
Using the with
Statement Beyond File Handling
The with
statement is not limited to file handling. It can also be used for managing resources like network connections and database connections.
import sqlite3
with sqlite3.connect('example.db') as connection:
cursor = connection.cursor()
cursor.execute('SELECT * FROM table_name')
In this example, the database connection is managed using the with
statement. Once the process is complete, the connection is automatically closed.
Best Practices
- Always use the
with
statement: Make it a habit to use thewith
statement when handling files or other resources. This prevents errors such as forgetting to close resources. - Write clean and concise code: Using the
with
statement helps keep your code simple and easy for others to understand.
7. Advanced Usage of the with
Statement in Python 3.3+
Since Python 3.3, the contextlib
module provides ExitStack
, which allows for more flexible resource management. This is particularly useful when handling a dynamic number of resources.
Using ExitStack
for Multiple File Operations
The following example demonstrates how to use ExitStack
to open multiple files simultaneously.
from contextlib import ExitStack
with ExitStack() as stack:
file1 = stack.enter_context(open('file1.txt', 'r'))
file2 = stack.enter_context(open('file2.txt', 'r'))
file3 = stack.enter_context(open('file3.txt', 'r'))
# Read the contents of each file
content1 = file1.read()
content2 = file2.read()
content3 = file3.read()
print(content1, content2, content3)
This method is useful when the number of resources may vary dynamically or when managing multiple types of resources together.
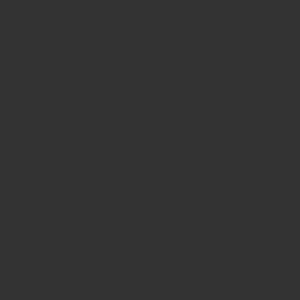
8. Enhancements to the with
Statement in Python 3.9+
In Python 3.9 and later, the with
statement has been further enhanced, making it easier to manage multiple context managers in a single line.
Using Tuples with the with
Statement
Starting from Python 3.9, multiple objects can be handled in a tuple-like structure within a single with
statement. The following example demonstrates this new syntax.
with (open('file1.txt', 'r') as file1,
open('file2.txt', 'r') as file2,
open('file3.txt', 'r') as file3):
content1 = file1.read()
content2 = file2.read()
content3 = file3.read()
print(content1, content2, content3)
This approach allows for a more compact syntax, improving code readability.
9. Precautions When Using the with
Statement
While the with
statement is highly useful and offers many advantages, there are some important points to consider.
Key Considerations When Using the with
Statement
- Combining with Exception Handling: If an exception occurs within a
with
block, the resource is still released automatically. However, using exception handling blocks improves code reliability. - Resource Compatibility: The
with
statement can be used with resources other than files, but only if they support context managers. If a resource does not support context managers, thewith
statement cannot be used.
10. Conclusion
The Python with
statement is a powerful tool for simplifying resource management, improving code safety, and enhancing readability. It is particularly useful for handling file operations, network connections, and other scenarios that require opening and closing resources.
- Code Simplification: The
with
statement automates resource closing, keeping code clean and concise. - Error Prevention: It helps prevent errors caused by forgetting to close resources, improving code reliability.
- Enhanced Functionality in Newer Python Versions: The
with
statement has been further enhanced in Python 3.3+ and 3.9+, allowing for more flexible and efficient resource management.
By actively utilizing the with
statement, you can improve your code quality and make your Python programming more efficient. Take this opportunity to master the with
statement and elevate your Python skills!