1. Basics of Python for
Loop
What is a for
Loop?
The for
loop is one of the most fundamental looping structures in Python. It is used to iterate over a specified range or sequence, processing each element one by one. This loop is commonly used to handle elements in data types such as lists, tuples, and strings.
Basic Syntax
The basic syntax of the Python for
loop is as follows:
for variable in sequence:
code to execute
In this syntax, each element in the sequence
is assigned to the variable
one by one, and the code inside the loop is executed repeatedly.
Example Using range()
with a for
Loop
for i in range(5):
print(i)
In this example, range(5)
generates integers from 0 to 4, assigning them to i
one at a time and printing each value.
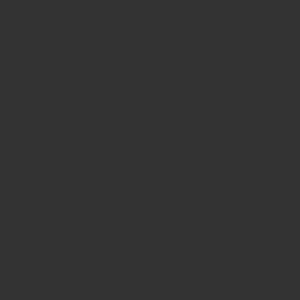
2. Iterating Over Different Data Types with for
Loops
Iterating Over Lists
A list is a data structure that holds multiple elements. Using a for
loop, you can iterate through each element in the list sequentially.
my_list = [1, 2, 3, 4, 5]
for value in my_list:
print(value)
This code extracts each element from the list and prints it.
Iterating Over Tuples
Like lists, tuples can also store multiple elements, and you can iterate through them using a for
loop.
my_tuple = (1, 2, 3)
for value in my_tuple:
print(value)
Iterating Over Dictionaries
Dictionaries store data in key-value pairs. To iterate over keys and values, use the items()
method.
my_dict = {"apple": "apple", "banana": "banana", "orange": "orange"}
for key, value in my_dict.items():
print(f"Key: {key}, Value: {value}")
Iterating Over Sets
A set is a collection of unique elements. You can iterate over its elements using a for
loop.
my_set = {"apple", "banana", "orange"}
for fruit in my_set:
print(fruit)
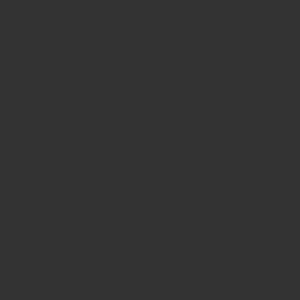
3. Controlling the for
Loop
Breaking a Loop with break
The break
statement is used to exit a loop when a specific condition is met.
for num in range(10):
if num == 5:
break
print(num)
In this example, the loop terminates when num
reaches 5.
Skipping an Iteration with continue
The continue
statement is used to skip the current iteration and move to the next one.
for num in range(10):
if num % 2 == 0:
continue
print(num)
In this example, even numbers are skipped, and only odd numbers are printed.
4. Nested for
Loops
Using a Nested Loop
By nesting for
loops, you can iterate over multiple sequences simultaneously.
for i in range(1, 4):
for j in range(1, 4):
print(i * j)
In this example, a nested loop is used to compute the product of numbers from 1 to 3.
Using a Triple Nested Loop
Triple nested loops can be used for more complex data structures.
for i in range(1, 4):
for j in range(1, 4):
for k in range(1, 4):
print(i * j * k)
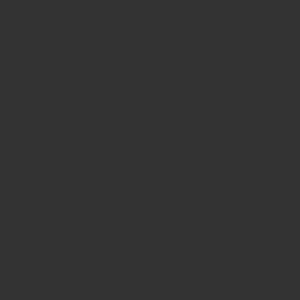
5. Iterating with enumerate()
How to Use enumerate()
The enumerate()
function allows you to retrieve both the index and the value of elements in a list or tuple.
my_list = [1, 2, 3, 4, 5]
for index, value in enumerate(my_list):
print(index, value)
In this example, both the index and value of each list element are printed.
6. Creating Lists Using List Comprehensions
Basic List Comprehension
List comprehensions allow you to create lists in a more concise and readable way.
my_list = [i * 2 for i in range(5)]
print(my_list) # [0, 2, 4, 6, 8]
In this example, the values from range()
are multiplied by 2 and stored in the list.
Conditional List Comprehension
By including an if
statement in a list comprehension, you can filter elements based on conditions.
my_list = [i for i in range(10) if i % 2 == 0]
print(my_list) # [0, 2, 4, 6, 8]
7. Practical Examples
Processing Files
The for
loop is commonly used to read and process files line by line.
with open('sample.txt', 'r') as f:
for line in f:
print(line.rstrip())
This code reads each line of the sample.txt
file and prints it after removing trailing spaces.
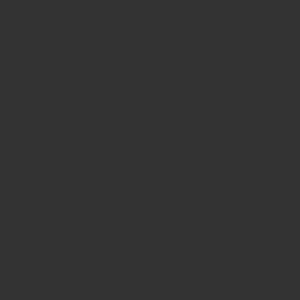
8. Tips and Best Practices for Using for
Loops
Writing Efficient Loops
When using for
loops, it is important to write efficient code by avoiding unnecessary computations. For example, instead of performing the same calculation repeatedly inside the loop, compute it once before the loop begins.
Writing Readable Code
Nesting too many for
loops can reduce code readability. Try to keep the structure as simple as possible. Also, using meaningful variable names helps clarify the purpose of the code.
Common Pitfalls
When modifying a list inside a for
loop, be cautious as adding or removing elements may cause unexpected behavior. Additionally, when processing large amounts of data in a loop, consider the execution time to optimize performance.
9. Summary
In this article, we covered everything from the basics to advanced techniques of Python’s for
loop. The for
loop is an essential tool for performing repetitive tasks and can be used in many different scenarios. By understanding its usage and control mechanisms, you can write more efficient and readable code.